Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial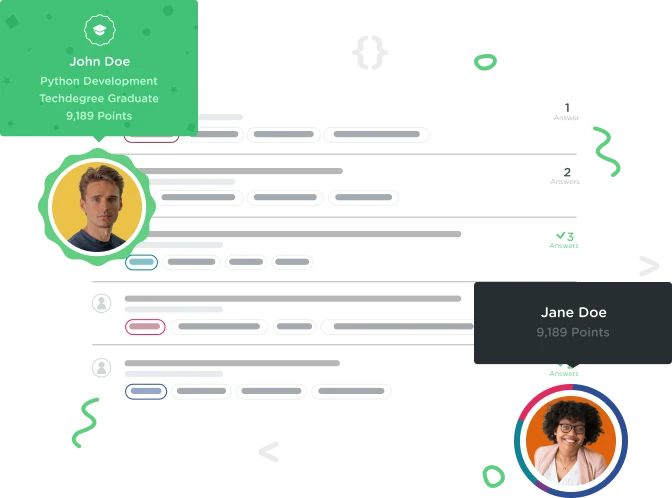

mohamed hassan
2,079 Pointshow to add a property to a subclass ?
I have tried to solve the challenge as the same way of the video however it keeps returning errors after reading some things about inheritance and initializers and how to add into the sub class it never used the override , I tried do it first in the play ground and it went through however here it is nor I need help , I don't know what exactly I did wrong here is my answer In the editor, I have provided a class named Vehicle.
Your task is to create a subclass of Vehicle, named Car, that adds an additional stored property numberOfSeats of type Int with a default value of 4.
Once you've implemented the Car class, create an instance and assign it to a constant named someCar.
class Vehicle {
var numberOfDoors: Int
var numberOfWheels: Int
init(withDoors doors: Int, andWheels wheels: Int) {
self.numberOfDoors = doors
self.numberOfWheels = wheels
}
class Car: Vehicle {
var numberOfSeats : Int
init (withDoors doors: Int, andWheels wheels: Int, numberOfSeats: Int) {
super.init(withDoors: doors, andWheels: wheels)
self.numberOfSeats = 4
}
}
let someCar = Car(withDoors: 4, andWheels: 4, numberOfSeats: 4)
// Enter your code below
1 Answer

Jeremy Conley
16,657 PointsSo since the default value of the new stored property is 4, you don't need to create a new init method for the subclass. Create your new car using the init method from the parent class.
class Vehicle {
var numberOfDoors: Int
var numberOfWheels: Int
init(withDoors doors: Int, andWheels wheels: Int) {
self.numberOfDoors = doors
self.numberOfWheels = wheels
}
}
// Enter your code below
class Car: Vehicle {
var numberOfSeats = 4
}
let someCar = Car(withDoors: 4, andWheels: 4)
Jeremy Conley
16,657 PointsJeremy Conley
16,657 PointsHowever if you did want to initialize your subclass with a certain number of seats, it would look like this