Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial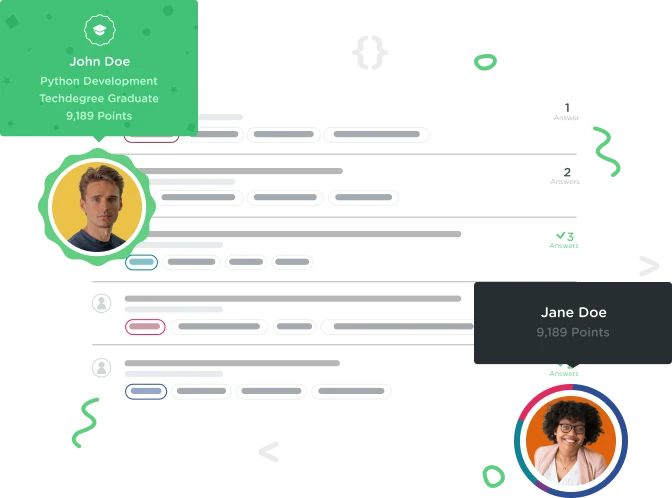
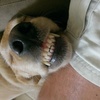
Herb Bresnan
10,658 PointsHow to add commas between list items?
I am having trouble with the first challenge given by Kenneth Love. He asks to print the list with commas between each item. I am stumped. I know the same question has been asked, but the answers do not seem to work. This is the original code:
shopping_list = list()
print("What should we pick up at the sore today? ")
print("Enter 'DONE' to stop adding items. ")
while True:
new_item = input("> ")
if new_item == "DONE":
break
shopping_list.append(new_item)
print("Added: List has {} items.".format(len(shopping_list)))
continue
print("Here's your list:")
for item in shopping_list:
print(item)
by concatenating the comma: print(item + ',') adds a comma AFTER the items which means there is a comma where it should not be, after the last item. There should be a simple solution to this but I am not seeing it.
4 Answers

Dan Johnson
40,533 PointsBasically you just need to determine if you're at the end of a list in some way to skip the last comma. You could ignore the last element in the loop:
report = ""
for item in shopping_list[:-1]:
report += item + ", "
print(report + shopping_list[-1])
Or check if the you're at the last element:
report = ""
num_items = len(shopping_list)
for i in range(num_items):
if i != num_items - 1:
report += shopping_list[i] + ", "
else:
report += shopping_list[i]
print(report)
If you're familiar with unpacking lists this functionality is built into the print function:
print(*shopping_list, sep=", ", end=" ")

Luiz Edgard Oliveira
1,524 PointsHello Herb,
Although your original question has been already extensively answered by Dan above, I'd like to pitch in with an alternative that seems more in line with what has been discussed in this Treehouse course so far. Dan's solution is comprehensive, but to a complete beginner like myself, it was a bit hard to understand at first. What I did was to attempt to complete the first challenge by using only keywords presented to us by Kenneth in the videos up to this point in the course.
In the original code, instead of:
print("Here's your list:")
for item in shopping_list:
print(item)
I used:
print("Here's your list:")
new_list = ', '.join(shopping_list)
print("{}".format(new_list))
This simpler solution seems to work nicely for the first challenge presented by Kenneth. If for some reason my solution isn't advisable, please let me know.

Russell Holz
4,811 PointsThank this answer actually makes sense to me, and is in line with the logic and methods presented thus far.
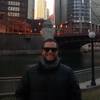
Andrew Lopes
3,190 PointsCan't I use just use this?
print(', '.join(shopping_list))
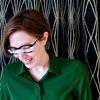
Vanessa Van Gilder
7,034 PointsThat's what I did, Andrew. It seems like the most concise way and in line with what Kenneth taught.
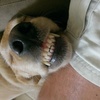
Herb Bresnan
10,658 PointsAnd the fog lifts. Thanks for your time.
Herb Bresnan
10,658 PointsHerb Bresnan
10,658 PointsDan, Thank you. This is the first answer I (mostly) understand. 3 short questions to clear it up. In the first block, is [:-1] the index? If so, why [-1]? And why is it preceded by a colon?
Also, do you know of a good resource to learn beginner Py to use along with Treehouse?
Dan Johnson
40,533 PointsDan Johnson
40,533 PointsList slicing has the following syntax:
my_list[start:end:steps]
So
my_list[:-1]
means, "Start from the beginning and go to the next to last element". Negative indexes start from the right, so -1 is the last element, -2 is the next to last element, and so on. You could also write it like this:my_list[0:-1]
.As for resources, the official site has a page listing a lot of guides: Beginner's Guide
For a paid option Safari Books has just about any tech book you could want and it's subscription based so you don't have to buy the books outright: Safari Books