Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial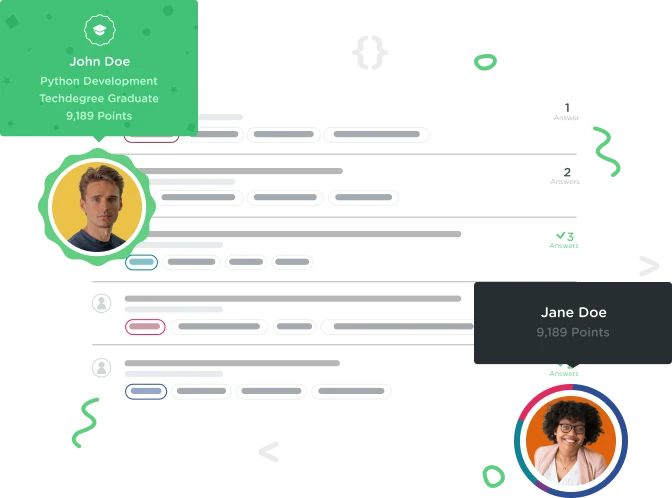
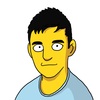
Sebastian Velandia
24,676 PointsHow to add different fields beetween Employees and customers?
In the video Hampton explained how to add inheritance for employees and Customers, both inherit from Account and both have the same fields
How can I set up different fields for Employees and Customers?
let's say I want to add the field "preferences" for customers but not for employees and "Hour rate" for employees but not for customers
I do not know how to do that. Thanks
1 Answer
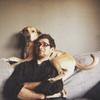
Joshua Shroy
9,943 PointsHey Sebastian,
If you need to add more fields (aka columns) to the database, you will first want to add them to the base model Account
. To do this, use can use the following command:
rails g migration add_preferences_and_hour_rate_to_account preference:string hour_rate:integer
Then you can create validations on each model to specify exactly which data will be used from the base model.
For example..
class Account < ActiveRecord::Base
end
class Employee < Account
validates_inclusion_of :hour_rate
validates_exclusion_of :preferences
end
class Customer < Account
validates_inclusion_of :preferences
validates_exclusion_of :hour_rate
end
Sebastian Velandia
24,676 PointsSebastian Velandia
24,676 PointsHo, Ok let me know if I understood, from the database point of view, I will have a table accounts with the columns for customers and employees? so when I create a new employee the columns for customers are going to be null in the data base? is that a good design pattern? is there another way to do it?
Joshua Shroy
9,943 PointsJoshua Shroy
9,943 PointsYou would actually have a table
accounts
with a column calledtype
which would store the class/model it's associated with. I'm sorry I forgot to mention in the post above that you would need to create the columntype
as a string in accounts:rails g migration add_type_to_accounts type:string
Let's say you created an employee with
Employee.create(name: "Charles")
, this record would be saved in theaccounts
table with:type = Employee
. This would allow you to fetch this record later on with something likeAccount.where(name: 'Charles').first
which will return a Employee object.It's a great design pattern when you have models in need of near identical fields such as the one above. If you feel like you will need more customized fields than you might want to start thinking about creating separate tables for Employees and Customers.
Hope that helps!
Sebastian Velandia
24,676 PointsSebastian Velandia
24,676 PointsThank you!, I think it's clearer to me now.. Thanks again =)
Joshua Shroy
9,943 PointsJoshua Shroy
9,943 PointsNo problem :)