Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial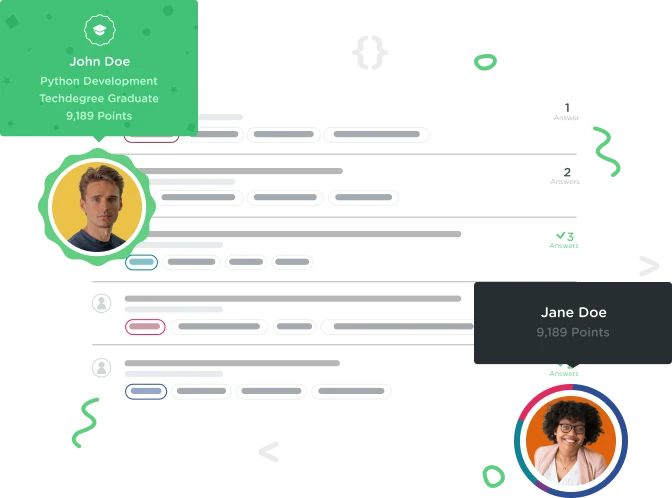

Primadonna Javier
iOS Development Techdegree Student 4,236 PointsHow to add "enter" event listener aside from clicking the button.
I am trying to apply what I learned from the Javascript and the DOM topic by building this simple to do list. My question is how do I add and event that when the user clicks "enter" key it will have the same function as clicking the button?
var myButton = document.querySelector('button.myB');
var myh1 = document.getElementById('title');
var input = document.getElementsByTagName('input')[0];
var myUl = document.getElementsByTagName('ul')[0];
myButton.addEventListener('click', function(){
var newLi = document.createElement('li');
newLi.textContent = input.value;
myUl.appendChild(newLi);
input.value = "";
});
myUl.addEventListener('click', function(e){
e.target.style.textDecoration="line-through";
});
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>repl.it</title>
<script src="index.js"></script>
<link href="index.css" rel="stylesheet" type="text/css" />
</head>
<body>
<h1 id="title">To do List</h1>
<ul>
</ul>
<input type="text" class="myInput">
<button class="myB">add item</button>
</body>
</html>
4 Answers
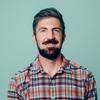
Ford Heacock
18,068 PointsHey Primadonna! For the enter key, you need to add a 'keyup' event listener, not a 'click'. You can then specify which exact key you are looking to listen to based on it's keycode. The enter key (a very common target) is 13. Check out http://keycode.io/ to learn the keycode numbers of other keyboard inputs. Working code below:
input.addEventListener('keyup',function(e){
if (e.keyCode === 13) {
alert('hi');
}
});
example on jsfiddle: https://jsfiddle.net/fordwh44/c4hrxzq6/

Primadonna Javier
iOS Development Techdegree Student 4,236 PointsHi Ford,
Thank you! you're amazing!
Regards, Primadonna
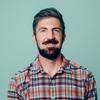
Ford Heacock
18,068 PointsOf course! Best of luck!
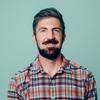
Ford Heacock
18,068 PointsHey Javier, adding multiple event listeners isn't a problem at all. Just add another one immediately after like so:
const input = document.querySelector('#myInput');
input.addEventListener('keyup',function(e){
if (e.keyCode === 13) {
alert('hi');
}
});
input.addEventListener('click',function(){
alert('you clicked me!');
});
Updated jsfiddle: https://jsfiddle.net/fordwh44/c4hrxzq6/

Primadonna Javier
iOS Development Techdegree Student 4,236 PointsPrimadonna Javier
iOS Development Techdegree Student 4,236 PointsHi Ford,
Thanks for the prompt response. What if I want two event listeners? I want to add "keyup" in the same function without removing click?
Thanks!