Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial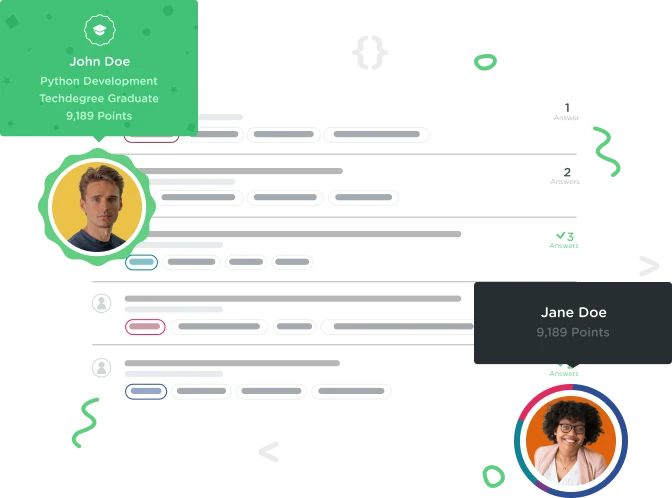
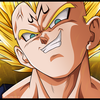
Sandro Meschiari
18,418 Pointshow to add location service to weather app
i would like to add the location service to my weather app as suggested on the extra challenge from Ben Jakuben but i got lost half way can anybody give me some tips?
2 Answers

deltaroom
10,229 PointsThis is an easy way to accomplish this. Adapt it to your Fragment or Activity, add the manifest permission and don't forget to update the location of your emulator (in case you are using one).
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />
public class MyFragment extends Fragment implements
ConnectionCallbacks, OnConnectionFailedListener, LocationListener {
private GoogleApiClient mGoogleApiClient;
private LocationRequest mLocationRequest;
public MyFragment() {
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
View view = //...
// your stuff
buildGoogleApiClient();
return view;
}
@Override
public void onStart() {
super.onStart();
mGoogleApiClient.connect();
}
protected synchronized void buildGoogleApiClient() {
mGoogleApiClient = new GoogleApiClient.Builder(getActivity())
.addConnectionCallbacks(this)
.addOnConnectionFailedListener(this)
.addApi(LocationServices.API)
.build();
}
protected void createLocationRequest() {
mLocationRequest = new LocationRequest();
mLocationRequest.setInterval(10000);
mLocationRequest.setFastestInterval(5000);
mLocationRequest.setPriority(LocationRequest.PRIORITY_HIGH_ACCURACY);
}
protected void startLocationUpdates() {
Log.i(TAG, "Started listening for location updates...");
LocationServices.FusedLocationApi.requestLocationUpdates(
mGoogleApiClient, mLocationRequest, this);
}
protected void stopLocationUpdates() {
Log.i(TAG, "Stopped listening for location updates");
LocationServices.FusedLocationApi.removeLocationUpdates(
mGoogleApiClient, this);
}
@Override
public void onConnected(Bundle connectionHint) {
Log.i(TAG, "Connected");
createLocationRequest();
startLocationUpdates();
}
@Override
public void onConnectionFailed(ConnectionResult result) {
Log.i(TAG, "Connection failed: ConnectionResult.getErrorCode() = " + result.getErrorCode());
}
@Override
public void onConnectionSuspended(int cause) {
Log.i(TAG, "Connection suspended");
mGoogleApiClient.connect();
}
@Override
public void onLocationChanged(Location location) {
double latitude = location.getLatitude();
double longitude = location.getLongitude();
stopLocationUpdates();
// do whatever you want to do with lat and long
}
}

Aishwarya Taneja
Courses Plus Student 66 PointsI didn't get how to make this work out.
Can you please share project included with location code.