Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial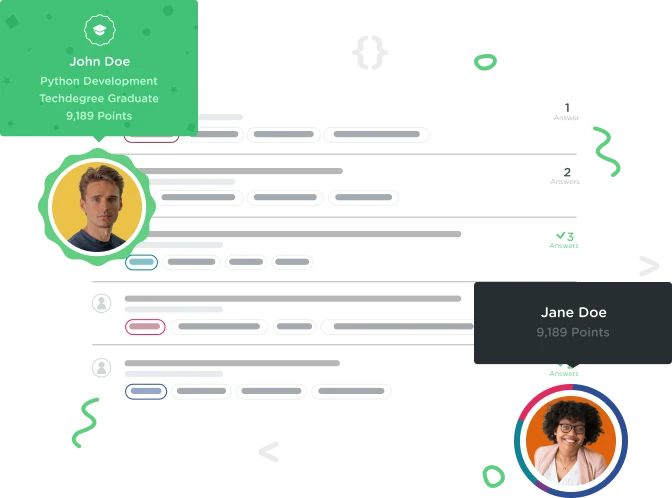
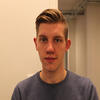
Coen van Campenhout
5,034 PointsHow to add multiple objects to a asp.net mvc form
So I am building an application where employees of a company have to be able to make orders for clients.
In my application I have a product class like this
public string Name { get; set; } public string Description { get; set; } public decimal Price { get; set; } public string Image { get; set; } public int Stock { get; set; } public int ID { get; set; }
public Product(string name, string description, string image, int stock, decimal price,int id)
{
this.Name = name;
this.Description = description;
this.Image = image;
this.Stock = stock;
this.Price = price;
this.ID = id;
}
the order class looks like this
public class Order { public List<Product> Products { get; set; } public int OrderID { get; } public int Price { get; set; } public Employee Salesman { get; set; } public DateTime OrderDate { get; set; } public int Payback { get; set; }
public Order(List<Product> products, int orderid, int price,Employee salesman,DateTime orderDate,int payback)
{
this.Products = products;
this.OrderID = orderid;
this.Price = price;
this.Salesman = salesman;
this.OrderDate = orderDate;
this.Payback = payback;
}
public Order()
{
}
in the order there is a list of products, however I just can't figure out how select all products in my databse and add the abilty to create a dropdown in my form where the user can just click on a product in the dropdown and it gets added to the product list any help would be much appreciated
Steven Parker
231,269 PointsSteven Parker
231,269 PointsYour questions are about database and form, but you didn't include those code portions.