Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial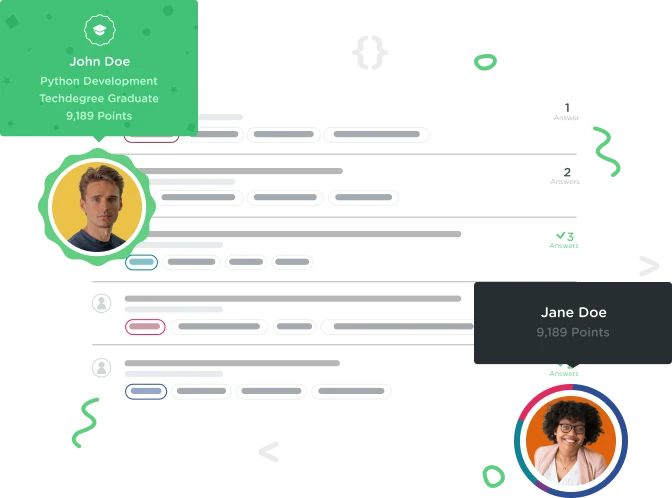
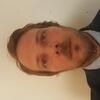
Jon Andreas Grenness
5,055 PointsHow to add the value in WeatherForecastId into the var weatherForecast
I have the following code:
using System; using System.IO; using System.Collections;
namespace Treehouse.CodeChallenges { public class Program { public static void Main(string[] arg) { }
public static WeatherForecast ParseWeatherForecast (string[] values)
{
WeatherForecast weatherForecast = new WeatherForecast();
using(var reader = new StreamReader(values))
{
string line = "";
while((line = reader.ReadLine()) != null)
{
var result = line.Split(',');
weatherForecast.Add(result);
}
}
return weatherForecast;
}
}
}
But I don't understand what I'm missing to assign the value in weatherForecastId into the var weatherForecast. Anyone see what I'm doing wrong?
using System;
using System.IO;
using System.Collections;
namespace Treehouse.CodeChallenges
{
public class Program
{
public static void Main(string[] arg)
{
}
public static WeatherForecast ParseWeatherForecast (string[] values)
{
WeatherForecast weatherForecast = new WeatherForecast();
using(var reader = new StreamReader(values))
{
string line = "";
while((line = reader.ReadLine()) != null)
{
var result = line.Split(',');
weatherForecast.Add(result);
}
}
return weatherForecast;
}
}
}
using System;
/* Sample CSV Data
weather_station_id,time_of_day,condition,temperature,precipitation_chance,precipitation_amount
HGKL8Q,06/11/2016 0:00,Rain,53,0.3,0.03
HGKL8Q,06/11/2016 6:00,Cloudy,56,0.08,0.01
HGKL8Q,06/11/2016 12:00,PartlyCloudy,70,0,0
HGKL8Q,06/11/2016 18:00,Sunny,76,0,0
HGKL8Q,06/11/2016 19:00,Clear,74,0,0
*/
namespace Treehouse.CodeChallenges
{
public class WeatherForecast
{
public string WeatherStationId { get; set; }
}
}
2 Answers
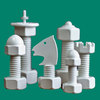
Steven Parker
231,268 PointsI'm not sure what you had in mind for creating a StreamReader (and the associated code using it). It's not asked for in the instructions, and in fact there's no data stream at all in this challenge.
For task 1, all you do is create the method, the variable inside it, assign a property, and return the variable. You've got 3 of the 4 done here, now just replace that entire "using" block with the one-line property assignment.
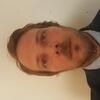
Jon Andreas Grenness
5,055 PointsI removed the using block, and tried adding weatherForecast.WeatherStationId; instead, but this causes an errormessage: "Program.cs(16,13): error CS0201: Only assignment, call, increment, decrement, await, and new object expressions can be used as a statement". I tried googling the errormessage, but I can't figure out what the problem is.
Might be that I'm just noobing :)
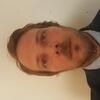
Jon Andreas Grenness
5,055 PointsI guess I was just noobing, solved it with the correct assignment:
weatherForecast.WeatherStationId = values[0];
Thanks for your help!