Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial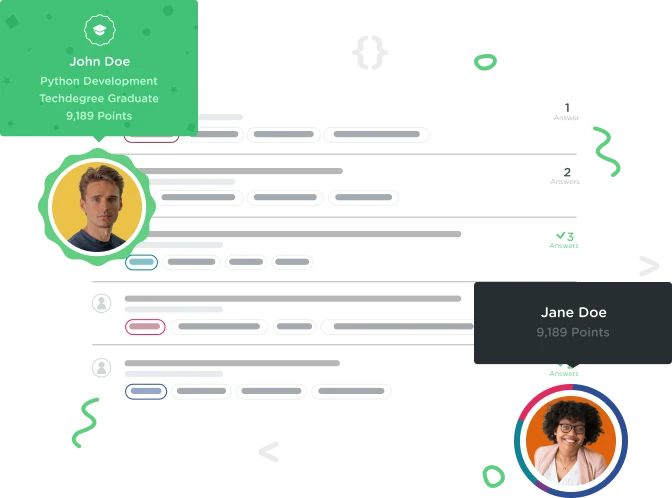
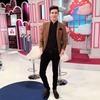
Christopher Evans
9,896 PointsHow to append class names to sibling elements
Really stuck on this one... Even the way the question is worded really confuses me. Not sure how to a) add a new class, or b) select an immediate previous sibling.
var list = document.getElementsByTagName('ul')[0];
list.addEventListener('click', function(e) {
if (e.target.tagName == 'BUTTON') {
append.className = "highlight";
}
});
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
</head>
<link rel="stylesheet" href="style.css" />
<body>
<section>
<h1>Making a Webpage Interactive</h1>
<p>Things to Learn</p>
<ul>
<li><p>Element Selection</p><button>Highlight</button></li>
<li><p>Events</p><button>Highlight</button></li>
<li><p>Event Listening</p><button>Highlight</button></li>
<li><p>DOM Traversal</p><button>Highlight</button></li>
</ul>
</section>
<script src="app.js"></script>
</body>
</html>
3 Answers
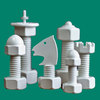
Steven Parker
231,269 PointsAssigning the name "highlight" to the className attribute of an element is a valid way to add the class name, but there's no element named "append" defined here.
Elements have an attribute called "previousElementSibling" that can be useful here for DOM traversal.
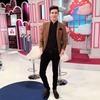
Christopher Evans
9,896 PointsSo what I tried to do was select the previous sibling element under a new variable, and then apply it to that variable. This also failed:
var list = document.getElementsByTagName('ul')[0];
list.addEventListener('click', function(e) {
if (e.target.tagName == 'BUTTON') {
let prev = list.previousElementSibling;
prev.className = "highlight";
}
});
Am I heading in the right direction or am I making it unnecessarily complicated?
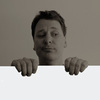
Sean T. Unwin
28,690 Points- You are being asked to select the previous element of the button that was clicked.
- To attach a CSS class to an element via code we use the classList property which has a method called, add()
We use e.target
to get the button then .previousElementSibling
then append the classList.add()
with the class name as the parameter for the add()
function.
Steven Parker
231,269 PointsSteven Parker
231,269 PointsYour second try is close, but the paragraph that you want to give the new class to is not a sibling of the list. It's a sibling of the button that was just pressed, and the tag name test just determined that the button is "e.target".