Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial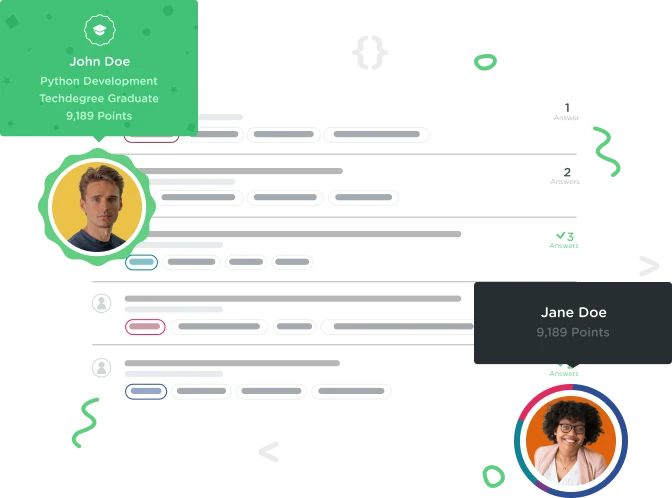

David Ton-Lai
3,268 PointsHow to append just the keys that intersect perfectly?
I'm having trouble appending only the keys that intersect with the set argument given.
COURSES = {
"Python Basics": {"Python", "functions", "variables",
"booleans", "integers", "floats",
"arrays", "strings", "exceptions",
"conditions", "input", "loops"},
"Java Basics": {"Java", "strings", "variables",
"input", "exceptions", "integers",
"booleans", "loops"},
"PHP Basics": {"PHP", "variables", "conditions",
"integers", "floats", "strings",
"booleans", "HTML"},
"Ruby Basics": {"Ruby", "strings", "floats",
"integers", "conditions",
"functions", "input"}
}
def covers1(setArg):
courseList = []
for key in COURSES.keys():
for value in COURSES.values():
#If the resulting intersecting length of setArg and the "value sets" is equal to the length of the setArg,
#then append that key to the list
if len(setArg & value) == len(setArg):
courseList.append(key)
return(courseList)
1 Answer

Jason Anello
Courses Plus Student 94,610 PointsHi David,
There's a few issues with the code.
The way that you have the nested for loops set up can lead to incorrect courses being appended and duplicates as well.
For each course in the outer loop, you're looping over all the topics for that course as well as all the other courses which isn't the correct logic.
A single loop is all that you need here where you loop over the course and its topics.
for key, value in COURSES.items():
The other issue is that you might have misinterpreted the instructions. You're not looking for a full intersection where nothing is left outside. It's ok if the course covers extra topics that weren't in the set passed in.
You should include the course if it covers at least 1 topic that was passed in.
For example, if the set {"Ruby", "loops"}
was passed in then both "Python Basics" and "Java Basics" should be included because they cover "loops" and "Ruby Basics" should be included because it covers "Ruby".
David Ton-Lai
3,268 PointsDavid Ton-Lai
3,268 PointsHi Jason,
Thanks, I don't know what I was thinking. I was probably looking at the code for too long and trying too hard to come up with the solution for it. Here is my code: