Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial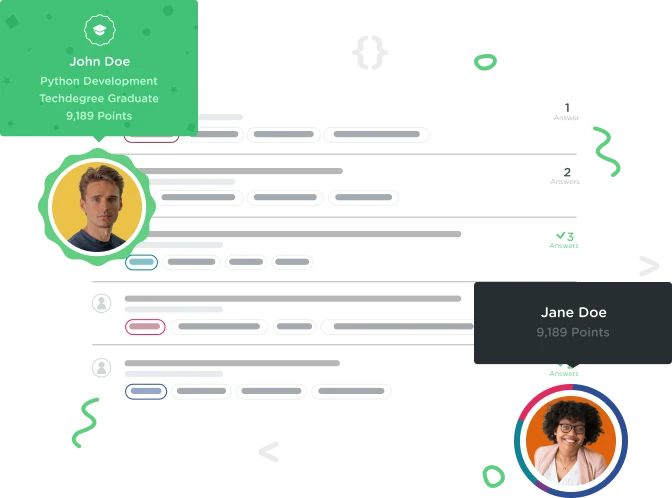

Yahya Lamont
Courses Plus Student 1,031 PointsHow to append values to Areay
How do append the values? could you point me in the right direction. Thanks in Advance!
var europeanCapitals: [String] = []
var asianCapitals: [String] = []
var otherCapitals: [String] = []
let world = [
"BEL": "Brussels",
"LIE": "Vaduz",
"BGR": "Sofia",
"USA": "Washington D.C.",
"MEX": "Mexico City",
"BRA": "Brasilia",
"IND": "New Delhi",
"VNM": "Hanoi"]
for (key, value) in world {
// Enter your code below
switch world {
case "BEL", "LIE", "BGR":
europeanCapitals.append()
case "IND", "VNM": asianCapitals.append()
default: otherCapitals.append()
}
// End code
}
2 Answers

Addison Francisco
9,561 PointsHello,
First of all, you have an issue with the switch statement within your for-in loop.
// Enter your code below
switch world { ...
Let’s take a quick look at this snippet regarding for-in loops and dictionaries
Each item in the dictionary is returned as a (key, value) tuple when the dictionary is iterated, and you can decompose the (key, value) tuple’s members as explicitly named constants for use within the body of the for-in loop.
Apple Docs: Control Flow
You are evaluating the world
dictionary with your current declaration. What you need to evaluate is the key(s) within this dictionary. Since this switch statement is within the loop that is iterating over the world
dictionary, you only need to evaluate the key of the current item
// Enter your code below
switch key { ...
Now, regarding your original question about what you need to append. It’s actually very easy.
You are very close with your current code. All that is missing is you are not passing in the value of the current dictionary item that you need to append to the array. To do that, we want to pass in the local constant of the for loop value
to the .append()
method. You’ll want to do this for every switch case
// Enter your code below
switch world {
case "BEL", "LIE", "BGR":
europeanCapitals.append(value)
case "IND", "VNM":
asianCapitals.append(value)
default:
otherCapitals.append(value)
}
Does this help you?

Chris White
2,308 PointsThere are two ways we can append to an array and it looks like you're halfway there using the append
method built into arrays, you just need to add the value within the method:
europeanCapitals.append(value)
We're just taking each value and appending it to the array through the dot notation syntax.
The other way we could do it is to concatenate the existing arrays with new ones we're creating each loop through:
europeanCapitals = europeanCapitals + [value]
One thing to note, we're being literal with value
, it's not a placeholder for our example code. We defined value
as a variable we're using in the for
loop.
for (key, value) in world { }
Any time we use value within that loop, we'll get the associated value for the current loop repetition.
You can do whichever makes the most sense to you, they do the same thing but the dot notation feels cleaner to me so it's what I originally used when doing this exercise.
Just don't use both, it's best to be consistent or it makes it more difficult for us to read at a glance:
switch key {
case "BEL", "LIE", "BGR":
europeanCapitals = europeanCapitals + [value]
case "IND", "VNM":
asianCapitals.append(value)
default:
otherCapitals.append(value)
}

Addison Francisco
9,561 PointsYou can also use this to achieve the same result
europeanCapitals += [value]
Mathew Tran
Courses Plus Student 10,205 PointsMathew Tran
Courses Plus Student 10,205 PointsYou are calling the append function, but you are using no arguments.
Here is a related example of what you are doing, but the with the
print
function instead.