Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial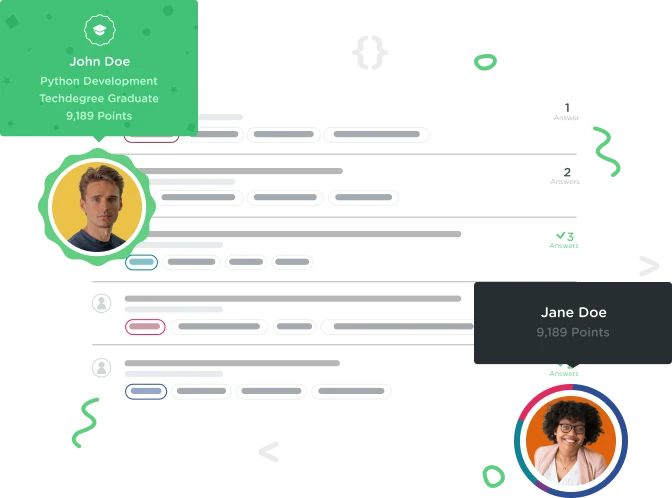
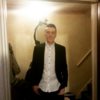
James Barrett
13,253 PointsHow to apply a class individually to each item?
Hi.
I have an array:
<?php
$listItems = array(
"Instagram",
"Github",
"Linkedin"
)
?>
I want to iterate over each item however they serve different purposes:
<?php foreach($listItems as $item) {
echo "<li><a href='https://instagram.com/jackson3195'><class='btn btn-default btn-lg'>" . $item . "</li>";
} ?>
All <li> items have a different class and need to be linked to different pages. I am not sure where to go from here?
Original HTML that is being attempted to be turnt to PHP:
<li>
<a href="https://instagram.com/jackson3195" class="btn btn-default btn-lg"><i class="fa fa-instagram fa-fw"></i> <span class="network-name">Instagram</span></a>
</li>
<li>
<a href="https://github.com/jackson3195" class="btn btn-default btn-lg"><i class="fa fa-github fa-fw"></i> <span class="network-name">Github</span></a>
</li>
<li>
<a href="https://www.linkedin.com/profile/view?id=AA4AABtkhjsBTlZ2ywxlxs3ZCaAbL3fY-1aAkd4&trk=send_invitation_success_message_name&goback=.npv_AAkAABtkhjsBFhFLyos*4ON*5Zp8chbhRd7omS92g_*1_*1_NAME*4SEARCH_mSum_*1_en*4US_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_tyah_*1_*1" class="btn btn-default btn-lg"><i class="fa fa-linkedin fa-fw"></i> <span class="network-name">Linkedin</span></a>
</li>
Thanks, James.
4 Answers
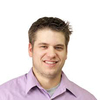
Kevin Korte
28,149 PointsOkay, how about we just extend what you're already doing. So let's add one more to your blog.php so it looks like this:
<?php
$pageTitle = "Jackson Jacob | Blog";
$pageHeading = "Blog Headline";
$pageSubHeading = "A blog by Jackson Jacob";
$is_blog = true;
include("inc/header.php");
?>
Note, just to clarify, I am setting $is_blog
the the boolean value of true, not a string. This makes it easier.
Now in you header code we worked on yesterday.
Let's just extend it with a simple if statement. On all other pages, you don't even have to set $is_blog to false, you can actually just ignore it.
What the code below is going to say is, if the variable is_blog is true, than display this, otherwise, display that. See below.
<?php
if($is_blog) {
echo "My big button code goes here";
} else {
$listItems = array(
"Instagram" => "https://instagram.com/jackson3195",
"Github" => "https://github.com/jackson3195",
"Linkedin" => "https://www.linkedin.com/profile/view?id=AA4AABtkhjsBTlZ2ywxlxs3ZCaAbL3fY-1aAkd4&trk=send_invitation_success_message_name&goback=.npv_AAkAABtkhjsBFhFLyos*4ON*5Zp8chbhRd7omS92g_*1_*1_NAME*4SEARCH_mSum_*1_en*4US_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_tyah_*1_*1"
);
foreach($listItems as $item => $url) {
echo "<li>";
echo "<a href='".$url."' class='btn btn-default btn-lg'><i class='fa fa-".strtolower($item)." fa-fw'></i><span class='network-name'>".$item."</a>";
echo "</li>";
};
};
That's all there is too it. You only have to set one variable, on time on the blog.php page. By default it will show your 3 social media links. I updated the snapshot to include similar code here: https://w.trhou.se/ofgb6839s3
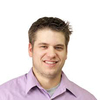
Kevin Korte
28,149 PointsHow about this:
<?php
$listItems = array(
"Instagram" => "https://instagram.com/jackson3195",
"Github" => "https://github.com/jackson3195",
"Linkedin" => "https://www.linkedin.com/profile/view?id=AA4AABtkhjsBTlZ2ywxlxs3ZCaAbL3fY-1aAkd4&trk=send_invitation_success_message_name&goback=.npv_AAkAABtkhjsBFhFLyos*4ON*5Zp8chbhRd7omS92g_*1_*1_NAME*4SEARCH_mSum_*1_en*4US_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_*1_tyah_*1_*1"
);
foreach($listItems as $item => $url) {
echo "<li>";
echo "<a href='".$url."' class='btn btn-default btn-lg'><i class='fa fa-".strtolower($item)." fa-fw'></i><span class='network-name'>".$item."</a>";
echo "</li>";
};
I think I hit everything, read it and let me know what doesn't make sense, otherwise check out the html here: http://port-80-duc9r3ugv6.treehouse-app.com/
Snapshot: https://w.trhou.se/j0iwm2c030
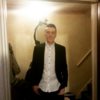
James Barrett
13,253 PointsAmazing! The code is so much cleaner. I understand the code... Just struggling to get to grips with the use of quotation marks throughout
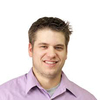
Kevin Korte
28,149 PointsHa, quotations are a pain, I had to do it twice to get it right, cause they are so easy to mess up. When it's narly like this, I build it out very slowly.
Just remember. Double quotes opens and closes a string to the php parser. The single quotes are technically just a string character, so in the final php, the double quotes are gone, and all that is left is the single quotes, and what is between them.
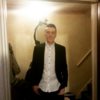
James Barrett
13,253 PointsI will make sure to read through it carefully, thanks again. Also another question, if I had another page with a slightly different header (so in my case, I have a blog page with header without the social media buttons; and just one completely different button there instead, how would I go about this without having to create a whole new PHP file?
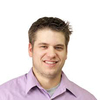
Kevin Korte
28,149 PointsAre the social media links in a header file, and you include the header file in your main files? Is that what you have going on?
And you want to either show these social media buttons, or something else, depending on the page the user is on?
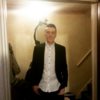
James Barrett
13,253 PointsYes, I have the social media links in the header file, using the foreach method which we have just discussed and they are included in each file. And also yes, I want to show either the social media buttons on the majority of pages and just one button on another page. If you would like me to share my code I would be more than happy to :-)
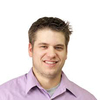
Kevin Korte
28,149 PointsSure, what does your code look like? The headers, and the two different page templates.
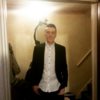
James Barrett
13,253 PointsIn case you do not receive notifications about new answers, I am just commenting here to show I have posted something new. :)
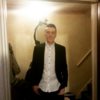
James Barrett
13,253 PointsCode for Blog.php:
<?php
$pageTitle = "Jackson Jacob | Blog";
$pageHeading = "Blog Headline";
$pageSubHeading = "A blog by Jackson Jacob";
include("inc/header.php");
?>
<!-- Page Content -->
<div class="container">
<div class="row">
<h1>Blog Headline</h1>
<p>Blog Snippet</p>
<div class="button-position">
<a href="blogentryone.php" class="button">Read More!</a>
</div>
</div>
<div class="row">
<h1>Blog Headline</h1>
<p>Blog Snippet</p>
<div class="button-position">
<a href="blogentrytwo.php" class="button">Read More!</a>
</div>
</div>
</div>
<!--Social Media Banner -->
<?php include("inc/banner.php"); ?>
<!-- Footer -->
<?php include("inc/footer.php"); ?>
<!-- jQuery -->
<script src="js/jquery.js"></script>
<!-- Bootstrap Core JavaScript -->
<script src="js/bootstrap.min.js"></script>
<!-- Google Analytics -->
<script>
(function(i, s, o, g, r, a, m) {
i['GoogleAnalyticsObject'] = r;
i[r] = i[r] || function() {
(i[r].q = i[r].q || []).push(arguments)
}, i[r].l = 1 * new Date();
a = s.createElement(o),
m = s.getElementsByTagName(o)[0];
a.async = 1;
a.src = g;
m.parentNode.insertBefore(a, m)
})(window, document, 'script', '//www.google-analytics.com/analytics.js', 'ga');
ga('create', 'UA-71099021-1', 'auto');
ga('send', 'pageview');
</script>
</body>
</html>
Header.php code: The same as stated above, except now with the foreeach loop
Essentially I just want one button to be displayed instead of three (as shown above) on the blog page, however from using the foreach loop three are going to be displayed... I was thinking perhaps using a $_GET on the URL, however I don't want to use URL variables.
Any help would be fantastic!
Thanks, James.
James Barrett
13,253 PointsJames Barrett
13,253 PointsAwesome! Only just got around to seeing this, thank you so much for your help :-)