Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial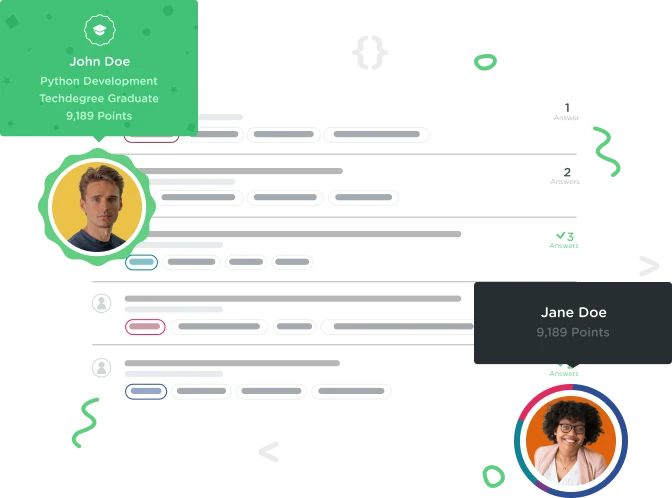

Kieran Barker
15,028 PointsHow to approach this additional practice: "Add a skill level to your exercises and display an exercise by skill level"?
On the "Daily Exercise Program" section of the PHP Basics course, Alena lists "Add a skill level to your exercises and display an exercise by skill level as well as day" under additional practice.
How would you approach this task? My thoughts were to add a variable, $skill
, and create three exercises for each day depending on the user's skill level: easy, medium, or hard. Then, in my if statement, I would do something like the following:
if ($day == 1 && $skill == 'Easy') {
echo $exercise1Easy;
}
If that makes sense.
But the issue I have is understanding how to dynamically set the skill level, like the date()
function getting the actual current date. In JavaScript, I would use an alert box or something to ask the user for their skill: Easy, Medium, Or Hard. But how can I make this work best for the user's skill, in PHP?
I hope this all makes sense! Thank you :-)
Ben Payne
1,464 PointsBen Payne
1,464 PointsHey Kieran,
You would capture the dynamic part or user input portion in a form on a web page. Then post that to a script, and handle the input.
You can use variable variables or an array to get the exercise. I would recommend an array for simplicity but I'll show you both:
Hopefully that helps.
-Ben