Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial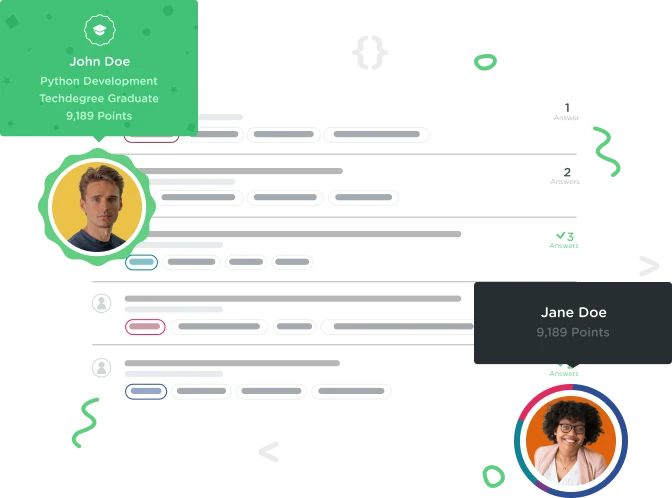

Nicolas Connor
3,410 PointsHow to assign a dictionary key value to a variable as a string
Hey guys the prompt for the question I am currently stuck on is : Wow, I just can't stump you! OK, two more to go. I think this one's my favorite, though. Create a function named most_courses that takes our good ol' teacher dictionary. most_courses should return the name of the teacher with the most courses. You might need to hold onto some sort of max count variable.
The error message I am currently getting is "Bummer! Couldn' find 'most_courses'". I'm guessing this is just a bug in the treehouse environment and it is being caused by a bug in my code.
The way I tried approaching this problem is by using a dictionary to store the prof that has taught the most courses. I know there are different ways of approaching this problem such as using ints and string to store the data instead of dicts but for the sake of learning, I'm using dicts instead.
I'm not sure where I am going wrong in my code but if I were to guess I would think it is on line 28 as I am probably assigning the courses that the professor taught instead of the name of the professor.
Would someone be able to confirm that my thery is correct and if so, is there a way that I could retrieve the value of one specific dict key so that I can store it temporarily in memory?
# The dictionary will look something like:
# {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Each key will be a Teacher and the value will be a list of courses.
#
# Your code goes below here.
def num_teachers(teacher_dict):
return len(teacher_dict)
def num_courses(teacher_dict):
courses_taught = 0
for teacher in teacher_dict:
courses_taught += len(teacher_dict[teacher])
return courses_taught
def courses(teacher_dict):
classes = []
for teacher in teacher_dict:
classes.extend(teacher_dict[teacher])
return classes
def most_courses(teacher_dict):
busiest_teacher = dict(name = None, courses_taught = 0)
for teacher in teacher_dict:
if len(teacher_dict[teacher]) > busiest_teacher[courses_taught]:
busiest_teacher[courses_taught] = len(teacher_dict[teacher])
busiest_teacher[name]=teacher # <---
return busiest_teacher[name]
2 Answers
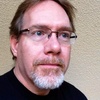
Chris Freeman
Treehouse Moderator 68,454 PointsThe issue isn't obvious at first glance. When using dict()
to create a dictionary the keywords will be used as literal strings for the dict keys. Beyond the initial dict()
defining busiest_teacher
, all subsequent literal key references 'courses_taught
' and 'name
' should include the quote marks. teacher
is a variable and does not need to be in quotes.
def most_courses(teacher_dict):
busiest_teacher = dict(name=None, courses_taught=0)
for teacher in teacher_dict:
if len(teacher_dict[teacher]) > busiest_teacher[courses_taught]: # <-- add quotes to courses_taught
busiest_teacher[courses_taught] = len(teacher_dict[teacher]) # <-- add quotes to courses_taught
busiest_teacher[name]=teacher # <-- add quotes to name
return busiest_teacher[name] # <-- add quotes to name
Post back if you have more questions! Good Luck!!
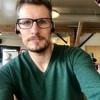
Josh Bennett
15,258 PointsI am confused...
Nicolas Connor
3,410 PointsNicolas Connor
3,410 PointsThank you! That makes total sense.