Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial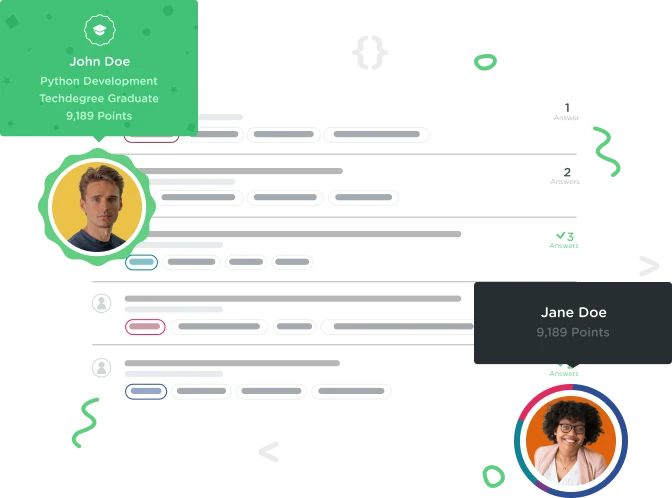

Ethan M
10,637 PointsHow to assign a user a post with Mongoose and Express
I am currently stuck on how I could assign a user a post when they create when in the website. So basically I want to have a logged in user create a resume and then have that resume assigned to that logged in user as a created_by in the schema. I kind of got it sorted but I don't know to create the resume and assign the logged in user to it.
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const ObjectId = Schema.ObjectId;
var moment = require('moment');
var id = require('../helpers/generator');
let beautifyUnique = require('mongoose-beautiful-unique-validation');
const ResumeSchema = new Schema({
_id: {
type: String,
default: id.generate()
},
title: {
type: String,
required: true
},
creator: {
type: ObjectId,
ref: "User"
},
description: {
type: String,
default: "No description provided."
},
company_reviews: {
name: {
type: String,
default: "RSW"
},
review: {
type: String,
default: "asodsodo"
},
rating: {
type: String,
default: "10/10"
}
},
employee_reviews: {
name: {
type: String,
default: "Doge Dog"
},
company: {
type: String,
default: "RSW"
},
review: {
type: String,
default: "blah blah"
},
rating: {
type: String,
default: "10/10"
}
},
createdAt: {
type: String,
default: moment(new Date()).format("MMM DD, YYYY") // "Sun, 3PM"
}
});
var Resume = mongoose.model('Resume', ResumeSchema);
module.exports = Resume;
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
var moment = require('moment');
var bcrypt = require('bcrypt');
var id = require('../../helpers/generator');
let beautifyUnique = require('mongoose-beautiful-unique-validation');
const UserSchema = new Schema({
_id: {
type: String,
default: id.generate()
},
firstName: {
type: String,
required: true
},
lastName: {
type: String,
required: true
},
accountType: {
type: String,
enum: ['Alphaneer', 'Administrator', 'Support', 'PRO'],
default: 'Alphaneer'
},
email: {
type: String,
required: true,
trim: true
},
username: {
type: String,
required: true,
trim: true,
unique: true
},
bio: {
type: String,
default: "No bio provided."
},
password: {
type: String,
required: true
},
createdAt: {
type: String,
default: moment(new Date()).format("MMM DD, YYYY") // "Sun, 3PM 17"
}
});
// authenticate input against content from database. BLOODY HELL ITS A MESS
UserSchema.statics.authenticate = function(email, password, callback) {
User.findOne({
email: email
})
.exec(function(err, user) {
if (err) {
return callback(err);
}
bcrypt.compare(password, user.password, function(err, result) {
if (result === true) {
return callback(null, user)
} else {
return callback();
}
})
})
}
UserSchema.pre('save', function(next) {
var user = this;
bcrypt.hash(user.password, 10, function(err, hash) {
if (err) throw err;
user.password = hash;
console.log("LOG: Password hashed & user saved.");
next();
});
});
var User = mongoose.model('User', UserSchema);
module.exports = User;
And I want to create a Resume as the logged in user here
// POST /dashboard/resume/create
router.post('/resume/create', (req, res, next) => {
Resume.create(req.body, (err, resume) => {
if (err) {
var err = new Error("Error:" + err);
err.status = 404;
next(err);
} else {
return res.redirect('/dashboard');
}
})
});
I also create a Resume when I register a user. Kind of a welcome thing but also have that resume referenced to the user
// POST /register
router.post('/', (req, res, next) => {
var data = {
firstName: req.body.fName,
lastName: req.body.lName,
username: req.body.username,
email: req.body.email,
password: req.body.password,
bio: req.body.bio
};
User.create(data, (err, user) => {
if (err) {
throw err;
}
var resume = {
title: "Welcome to NAME!"
}
Resume.create(resume, (err, resume) => {
if (err) throw err;
return res.redirect('/');
});
});
});
Any help is appreciated and I can also do some further explaining if it isn't making sense :)
3 Answers
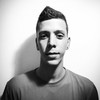
Jesus Mendoza
23,289 PointsHey Ethan,
In your userSchema you have to add a reference to the posts the user has created, you can do it with the following code:
posts: [
{
type: mongoose.Schema.Types.ObjectId,
ref: 'Post'
}
]
You also need to to add a reference to an user in each post you create, to assign ownership:
author: [
{
type: mongoose.Schema.Types.ObjectId,
ref: 'User'
}
]
Now, everytime you create a new post, you assign it an user
// We find the current logged user in our database
User.findById(req.user.id, (err, user) => {
if (err) throw new Error(err);
// We create an object containing the data from our post request
const newPost = {
title: req.body.title,
content: req.body.content,
// in the author field we add our current user id as a reference
author: req.user._id
};
// we create our new post in our database
Post.create(newPost, (err, post) => {
if (err) {
res.redirect('/');
throw new Error(err);
}
// we insert our newpost in our posts field corresponding to the user we found in our database call
user.posts.push(newPost);
// we save our user with our new data (our new post).
user.save((err) => {
return res.redirect(`/posts/${post.id}`);
});
})
});
Now you have access to the post author using
post.author.id
post.author.username
etc.
and now you can find all posts corresponding to each user using the populate method
// this will populate the posts field in our userSchema (which contain the id references to our posts)
User.findById(req.user.id).populate('posts').exec((err, user) => {
console.log(user.posts);
})

Ethan M
10,637 PointsThank you!

Jiel Selmanovski
5,535 PointsVery helpful! Thank you for the answer!