Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial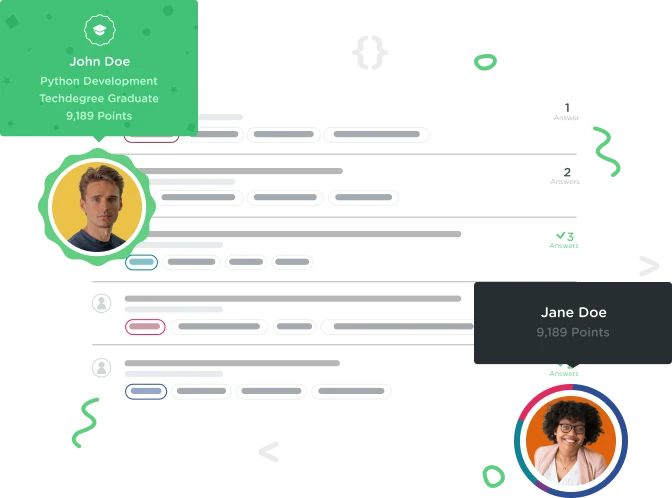
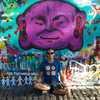
Andrew Stelmach
12,583 PointsHow to assign 'users' to 'groups', Associations etc
Ok, so I've got a User model and a Group model.
User belongs_to :group
Group has_many :users, dependent: :destroy
Rather than CREATE a User from within a group, I want to create Groups (easy), and ASSIGN Users to Groups.
How do I do that?
1 Answer
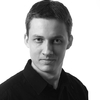
Maciej Czuchnowski
36,441 PointsI'm not 100% sure what your question is exactly, but:
1) Don't destroy the users along with the group! What if they want to join another group in the future when their current group is destroyed? Unless this is intended behavior.
2) What's your schema for groups and users? I want to show you a few examples of how to assign things both ways, if that is what you're asking ;)
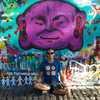
Andrew Stelmach
12,583 PointsGood point on no.1 - it was a brainfart.
create_table "groups", force: :cascade do |t|
t.text "description"
t.string "level"
t.datetime "created_at", null: false
t.datetime "updated_at", null: false
end
create_table "users", force: :cascade do |t|
t.string "email", null: false
t.string "crypted_password"
t.string "salt"
t.datetime "created_at"
t.datetime "updated_at"
t.string "name"
end
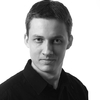
Maciej Czuchnowski
36,441 PointsAlright, first you need to add group_id (integer, preferably with index) field to your users to be able to actually assign them to groups.
has_many and belongs_to basically give you additional methods that do database queries for you. So go to the console and try this:
So when you create a group, you can access all its assigned users like this:
Group.first.users
This will return an empty array at first if no users are assigned to that group. User.first.group should also give you some empty value (nil), since that user does has his group_id set to nil.
Now you can assign users to groups using simple Ruby syntax:
group = Group.first
user = User.first
group.users << user
And the user gets his group_id set to this first group's id and when you call Group.first.users or User.first.group, they are no longer empty or nil.
If you really want to understand activerecord associations, you should read this book: http://easyactiverecord.com/
Andrew Stelmach
12,583 PointsAndrew Stelmach
12,583 PointsF*ck it, maybe I'll just use this? https://github.com/dwbutler/groupify
Some of that gem's features would be useful, actually, such as the ability to assign a User as a 'manager' of a group and be able to change the 'manager' also at a later date.