Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial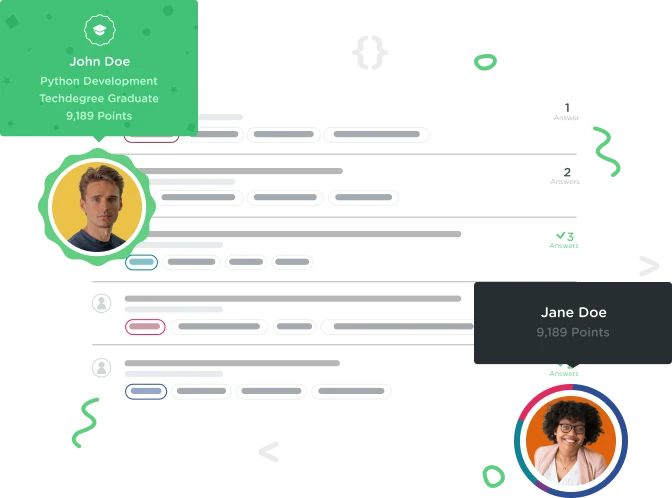

swiftfun
4,039 PointsHow to avoid repeating facts
How can I generate a random non-repeating number? To avoid showing the same fact twice (until all facts have been shown first)
The current code sometimes repeats number (hence repeating duplicate facts before going through the whole array:
import GameKit
struct QuoteProvider {
let quoteArray = [quote1, quote2, quote3, quote4, quote5]
func randomQuote() -> Quote {
let randomNumber = GKRandomSource.sharedRandom().nextInt(upperBound: quoteArray.count)
return quoteArray[randomNumber]
}
}
1 Answer
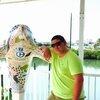
Ty Schenk
iOS Development Techdegree Graduate 16,269 PointsHello,
I think something like this would work great for what you are looking for. I made a few adjustments so please change those to reflect your code.
var quoteArray: [String] = ["quote1", "quote2", "quote3", "quote4", "quote5"]
var usedQuoteArray: [String] = []
func randomQuote() -> String {
if quoteArray.count == 0 {
resetQuotes()
}
// Get Random Number
let randomNumber = GKRandomSource.sharedRandom().nextInt(upperBound: quoteArray.count)
// Save Quote to constant
let quote = quoteArray[randomNumber]
// Add Quote tp usedQuoteArray
usedQuoteArray.append(quoteArray[randomNumber])
// Remove from Available
quoteArray.remove(at: randomNumber)
return quote
}
func resetQuotes() {
for quote in usedQuoteArray {
quoteArray.append(quote)
}
}