Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial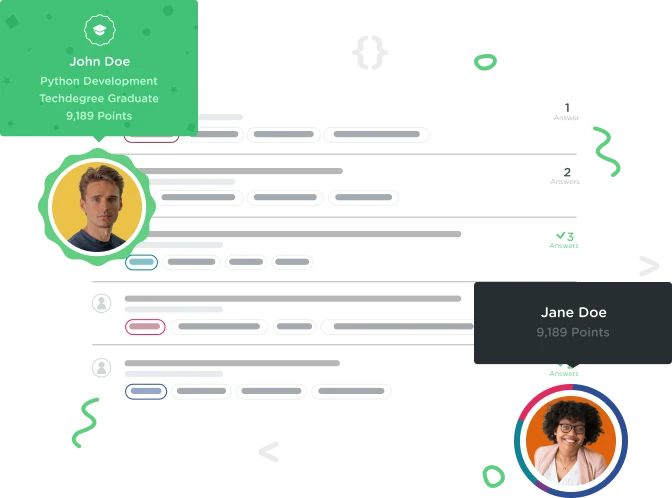

Tiago Ramos
2,380 PointsHow to calculate the average of tongue length?
Hi, I'm completely clueless on how to do my next step. I have 2 steps in mind: 1 - calculate the total of tongues length of all the frogs; 2 - divide the total by the number of frogs (frogs.length I guess)
But I don't know how to access the value of tongue lenght in the frogs[] array. Also should i use some .Net method to calculate the average?
Thanks a bunch mates! :)
namespace Treehouse.CodeChallenges
{
class FrogStats
{
public static double GetAverageTongueLength(Frog[] frogs)
{
for(i=0; i <= frogs.length; i++)
{
// int total_tongues_lenght =
}
}
}
}
namespace Treehouse.CodeChallenges
{
public class Frog
{
public int TongueLength { get; }
public Frog(int tongueLength)
{
TongueLength = tongueLength;
}
}
}
4 Answers
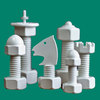
Steven Parker
231,268 PointsIt sounds like you have the right idea. To get to each tongue length, use your loop value (i) as an index into the array of frogs to get a single one, then access that property on it. You can then accumulate these values:
total_tongues_length += frogs[i].TongueLength;
Be sure to initialize the accumulator before the loop. Then after the loop just divide by the number of frogs and return the result.

Joe Beltramo
Courses Plus Student 22,191 PointsHello,
So you are going to want to define a variable outside of the for loop initially. It looks like you want to go with total_tongues_length
, so use that.
Next, the length property of an array is capitalized: frogs.Length
.
Within the loop you can simply, increment the total_tongue_length
variable by the frog's tongue length. In order to access the frog at a specific instance, if you recall from previous lessons, you can use an index in bracket notation, such as:
frogs[0]; // This will get you the first item of the frogs array
Luckily for us, in C#, you can instead of providing an index manually, supply the index with a variable (i in this case):
frogs[i];
Now that you have a hold of a Frog at the current index of i, you can use it as if you just had a variable assigned to a Frog. If we look in the Frog.cs file, we can see that the length of a tongue is stored as TongueLength
total_tongue_length += frogs[i].TongueLength;
Finally at the end of the method, ensure that you are returning a double
since the method does so, by converting your return statement like so:
return (double) total_tongue_length;
However, you need to return the average, so you want:
return (double) total_tongue_length / frogs.Length;
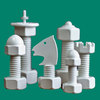
Steven Parker
231,268 PointsYou wouldn't need casting if you define "total_tongue_length" to be a double initially.

Joe Beltramo
Courses Plus Student 22,191 PointsI would define it as a double, but he has already defined it as an int and I didn't want to cause confusion. TongueLength is an int, he defined total_tongue_length as int, so work with ints, then cast at the end
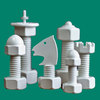
Steven Parker
231,268 PointsIt wasn't defined yet, though I did see "int" in a comment.
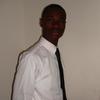
kekelidos
9,901 PointsTo get the average of tongue length, We need to add Frogs with different tongue lengths to the frogs Array. This means you need to create instances of Frog frogs[0] = new Frog(5); and so on. then you can loop over the frogs array, add up the tongueLength and divide it by frogs.length
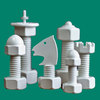
Steven Parker
231,268 PointsAll the frogs are in the array that the validator passes in to begin with.
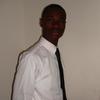
kekelidos
9,901 PointsPossible! But how could I tell if the argument of getAverageTongueLength is in fact containing the frogs. You are just assuming the frogs are in the array. Not a fact. There is nothing preventing me from passing in an empty array of Frog and the program will crash because of ArythmaticException(division by 0).
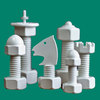
Steven Parker
231,268 PointsYou're right, and in a real program, some testing or exception handling would be a good idea. And these concepts are covered elsewhere.
But right here, the instructions only ask for a simple average calculation; so nothing that fancy is needed to pass the challenge.