Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial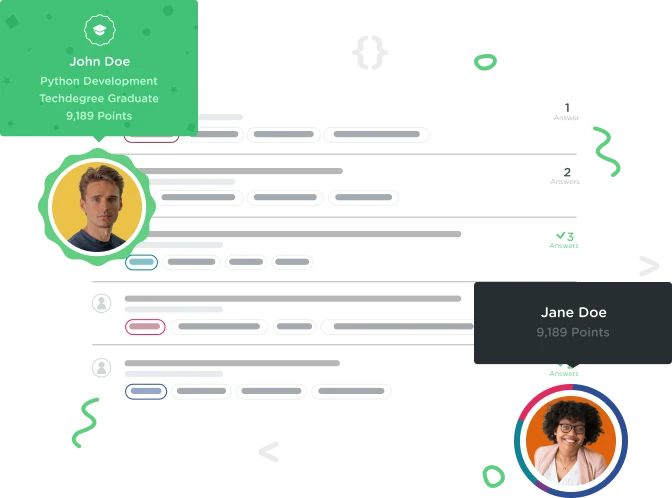

DV Shop
1,482 PointsHow to call a function and how to return value to a variable called.
Call the hello_student() function and save the return value to a variable called hello.
hello = "Hello"
def hello_student(name):
print(hello + name)
hello_student()
2 Answers

DV Shop
1,482 PointsSometimes, I tried many times to write this code and fix bug with my coding. Finally, I posted my Python Code in this forum in order to find out answer. Please help me and show me your code with this problem.
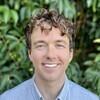
Asher Orr
Python Development Techdegree Graduate 9,408 PointsHi DV Shop!
Let's break it down by each task in the challenge:
Task 1 of 2: "Write a function called hello_student. This function should receive one argument, name. This function should return one value, the string 'Hello ' followed by the value of the name parameter."
I see that you defined the hello_student function properly
def hello_student(name):
To pass task 1, your hello_student function needs to: return the string "Hello" followed by the value of the name parameter.
When you click "Check work", the Treehouse Checker will run code like this:
hello_student("dv_shop")
To pass, it would see this returned:
Hello dv_shop
Let's get back to your code. On line 1, you are defining a variable outside of the function.
hello = "Hello"
# ^ this is the line I'm referring to
def hello_student(name):
print(hello + name)
hello_student()
I suggest removing this line. When Python goes into your hello_student function, it expects all variables to be defined within the function (this is not always the case, though. You can read more about this concept here.)
To pass the challenge, you just need the hello_student function to return the string "Hello " + the name variable.
You don't need to store any strings in a variable, then return that variable. Your function can be just 2 lines:
def hello_student(name):
#return the string "Hello " + the name variable
Task 2 of 2: Call the hello_student() function and save the return value to a variable called hello.
This line of code should start on line 4, unindented.
That way, Python will know your code is not part of the hello_student function. Like this:
def hello_student(name):
#return the string "Hello " + the name variable
#new code starts here
On line 4, make a variable called hello. Now call your hello_student function, and pass in whatever string you want as the name parameter:
hello = hello_student(your_string_value)
Whatever the function returns, it will be stored in the value "hello."
Does this answer your question?