Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial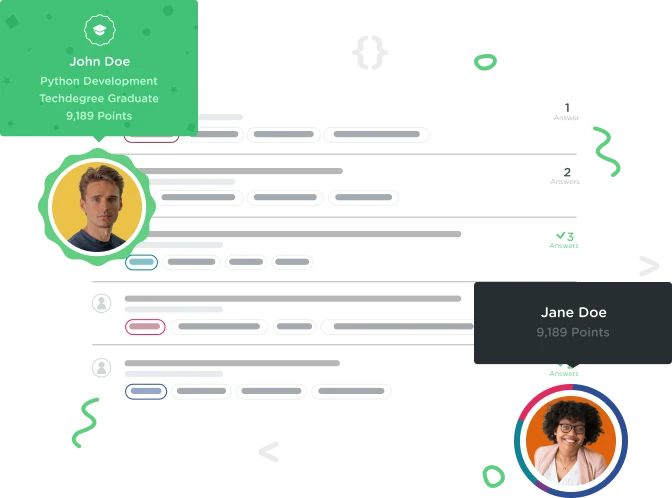

Yevhenii Zhdan
1,155 PointsHow to call a same method with different amount of parameters?
I can't call addItem method because in first time it is name and quantity and in second name only. I can't understand how to call the same method with different amount of parameters...
1 Answer

Ken Alger
Treehouse TeacherYevhenii;
What you seem to be referring to is known as method overloading. Let's create a quick example and then discuss it.
public void overloadingExample(String str) {
System.out.println(str);
}
public void overloadingExample(String str, int num) {
System.out.println(str + " " + num);
}
Hopefully what those two methods do makes some sense. As you can see, the method overloadingExample()
is overloaded based on the data type of arguments. What does that mean in terms of usage? Well, it allows us to call that method with either of the following examples and Java will not throw an error:
overloadingExample("example");
overloadingExample("another example", 2);
As one might expect, we would get the following data returned:
example
another example 2
Does that help at all? Java is pretty great at figuring out what to do with a method name based on the parameter you pass into the function, as long as you pass in the proper data types.
Post back if you have further questions.
Ken
Michael Makali'i Fernandez
8,090 PointsMichael Makali'i Fernandez
8,090 PointsI think what you want is to overload your methods. You need to have two methods with the same signature but different parameters.
Ex:
So you would call this method as