Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial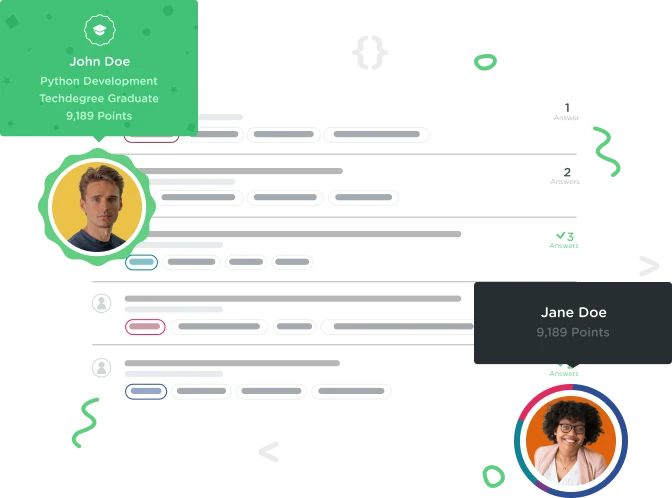

Gary Gibson
5,011 PointsHow to catch a value error on the bottom of this shopping list script?
Kenneth said to catch value errors at the bottom of this script. I've tried a few times but can't get it to work properly.
shopping_list = []
def show_help():
print("\nSeparate each item with a comma.")
print("""Type 'done' to quit, 'show' to see the curent list,
and 'help' to get this message as a reminder.""")
def show_list():
count = 1
for i in shopping_list:
print("{}: {}".format(count, i))
count += 1
print("Enter items for your shopping list.")
show_help()
while True:
new_stuff = input("Please enter an item. ")
if new_stuff == "quit":
print("\nHere's your list.")
show_list()
break
elif new_stuff == "help":
show_help()
continue
elif new_stuff == "show":
show_list()
continue
else:
new_list = new_stuff.split(",")
index = input("If you want to add this to a certain spot, enter the appropriate number. Or juts hit 'enter' to add it to the end of the list. Currently {} items in the list".format(len(shopping_list)))
if index:
spot = int(index) - 1
for item in new_list:
shopping_list.insert(spot, item.strip())
spot += 1
else:
for item in new_list:
shopping_list.append(item.strip())
6 Answers
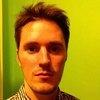
Brendan Whiting
Front End Web Development Techdegree Graduate 84,736 PointsWhat video or challenge is this? I suspect he wants you to use a try/except block: https://docs.python.org/2/tutorial/errors.html

Gary Gibson
5,011 PointsI didn't include it here.
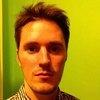
Brendan Whiting
Front End Web Development Techdegree Graduate 84,736 PointsCan you post the version that includes it?

Gary Gibson
5,011 Pointsshopping_list = []
def show_help():
print("\nSeparate each item with a comma.")
print("""Type 'done' to quit, 'show' to see the curent list,
and 'help' to get this message as a reminder.""")
def show_list():
count = 1
for i in shopping_list:
print("{}: {}".format(count, i))
count += 1
print("Enter items for your shopping list.")
show_help()
while True:
new_stuff = input("Please enter an item. ")
if new_stuff == "quit":
print("\nHere's your list.")
show_list()
break
elif new_stuff == "help":
show_help()
continue
elif new_stuff == "show":
show_list()
continue
else:
new_list = new_stuff.split(",")
try:
index = input("If you want to add this to a certain spot, enter the appropriate number. Or juts hit 'enter' to add it to the end of the list. Currently {} items in the list".format(len(shopping_list)))
except ValueError:
print("Please enter a number.")
else:
if index:
spot = int(index) - 1
for item in new_list:
shopping_list.insert(spot, item.strip())
spot += 1
else:
for item in new_list:
shopping_list.append(item.strip())
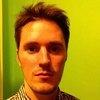
Brendan Whiting
Front End Web Development Techdegree Graduate 84,736 PointsAnd which challenge or video is this for?
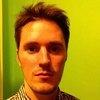
Brendan Whiting
Front End Web Development Techdegree Graduate 84,736 PointsHere is my version:
shopping_list = []
def remove_item(idx):
index = idx - 1
item = shopping_list.pop(index)
print("Remove {}.".format(item))
def show_help():
print("\nSeparate each item with a comma.")
print("Type DONE to quit, SHOW to see the current list, and HELP to get this message, REMOVE to delete an item")
def show_list():
count = 1
for item in shopping_list:
print("{}: {}".format(count, item))
count += 1
print("Give me a list of things you want to shop for.")
show_help()
while True:
new_stuff = input("> ")
if new_stuff == "DONE":
print("\nHere's our list:")
show_list()
break
elif new_stuff == "HELP":
show_help()
continue
elif new_stuff == "SHOW":
show_list()
continue
elif new_stuff == "REMOVE":
idx = input("Which item? Tell me the number. ")
remove_item(int(idx))
else:
new_list = new_stuff.split(",")
index = input("Add this at a certain spot? Press enter for the end of the list, " "or give me a number. Currently {} items in the list. ".format(len(shopping_list)))
while True: #making a loop, so they can keep trying til they get it right
if len(index): ## if they didn't just hit "enter"
try:
int(index) ## if index can be converted to an int, it will keep going, otherwise it raise a ValueError and jump to the except block
spot = int(index) - 1
for item in new_list:
shopping_list.insert(spot, item.strip())
spot += 1
break ##exiting this while loop because we succeeded this task
except ValueError:
index = input("That wasn't a number. Try giving me a number again for a spot, or press enter for the end of the list")
## in this case it's going to loop back again to line 41, test to see if they hit enter and test to see if it's an int
else: ## if index doesn't have a length, and they just hit "enter" we put their items at the end
for item in new_list:
shopping_list.append(item.strip())
break

Gary Gibson
5,011 PointsI don't know what I did, but now this code works:
shopping_list = []
def show_help():
print("\nSeparate each item with a comma.")
print("""Type 'done' to quit, 'show' to see the curent list,
and 'help' to get this message as a reminder.""")
def show_list():
count = 1
for i in shopping_list:
print("{}: {}".format(count, i))
count += 1
print("Enter items for your shopping list.")
show_help()
while True:
new_stuff = input("Please enter an item. ")
if new_stuff == "quit":
print("\nHere's your list.")
show_list()
break
elif new_stuff == "help":
show_help()
continue
elif new_stuff == "show":
show_list()
continue
elif new_stuff == "":
print("Nothing? Are you sure?")
else:
new_list = new_stuff.split(",")
try:
index = input("If you want to add this to a certain spot, enter the appropriate number. Or juts hit 'enter' to add it to the end of the list. Currently {} items in the list".format(len(shopping_list)))
except ValueError:
print("Please enter a number.")
else:
if index:
spot = int(index) - 1
for item in new_list:
shopping_list.insert(spot, item.strip())
spot += 1
else:
for item in new_list:
shopping_list.append(item.strip())
Gary Gibson
5,011 PointsGary Gibson
5,011 PointsI know, but my use of it is not doing what I want
Brendan Whiting
Front End Web Development Techdegree Graduate 84,736 PointsBrendan Whiting
Front End Web Development Techdegree Graduate 84,736 PointsWhere are you using it? I don't see.