Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial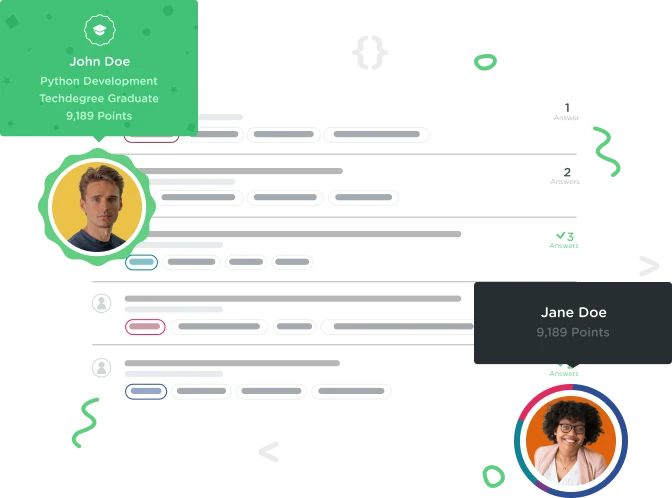
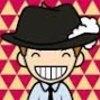
Aaron Selonke
10,323 PointsHow to chain event handlers
Trying to understand why the event handlers are chained up that way. Are these two blocks of code exactly the same?
$("#password").focus(passwordEvent).keyup(passwordEvent);
$("#password").focus(passwordEvent);
$("#password").keyup(passwordEvent);
3 Answers
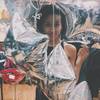
Akosua Kernizan
19,994 PointsBoth blocks of code will give you the same result, but in an effort to keep code DRY (don't repeat yourself) you would write it the chained way.

William Hirsh
12,712 PointsAre you not repeating yourself when you called and executed the passwordEvent function twice in the same statement. When I followed the jQuery API documentation before watching the video I obtained this solution that I believe works a bit better. I was very surprised that Andrew Chalkley skipped going into the keyUp() documentation. I always try the perform step before watching the video because Andrew taught the importance of using the jQuery API documentation and he gave links to the new methods needed in the Teacher's Notes below.
//Hide hints
$("form span").hide();
//When event happens on password input
$("#password").focus(function () {
$(this).keyup(function (){
//Find out if password is valid
if ( $(this).val().length > 8) {
//Hide hint if valid
$(this).next().hide();
} else {
//else show hint
$(this).next().show();
}
});
});
In this solution, within the focus binding, You are calling an anonymous function. Inside is a binding of the keyup event that calls another (different) anonymous function after the field is focused. Instead of calling and executing the same function statements twice on keyup, you are trickling down to a single anonymous function inside the keyup event. I decided to try and explain what I was doing in case other students want to follow along. If I made any mistakes please correct me on that.

Jason Anello
Courses Plus Student 94,610 PointsHi William,
The passwordEvent function isn't being called yet in the code posted in the question. The code sets up both a focus and a keyup handler and a reference to the passwordEvent function is passed in as an argument. If the element either receives focus OR there's a keyup event for that element then the passwordEvent function will be called and executed in response to either of those events.
Your code is doing something different from this.
Your code first attaches a focus handler to the element and that's it. The anonymous function passed in will run as soon as the element receives focus. When the element does receive focus then a keyup handler will be attached to the element. The problem is that your code will attach a keyup handler every time the element receives focus. This means the code to check the password could be run multiple times in response to a single keyup event.

William Hirsh
12,712 PointsThanks for letting me know. I was wondering why the other way was preferred. You see a similar process, to what I did, in the jQuery API documentation for the keyup keyboard event.