Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial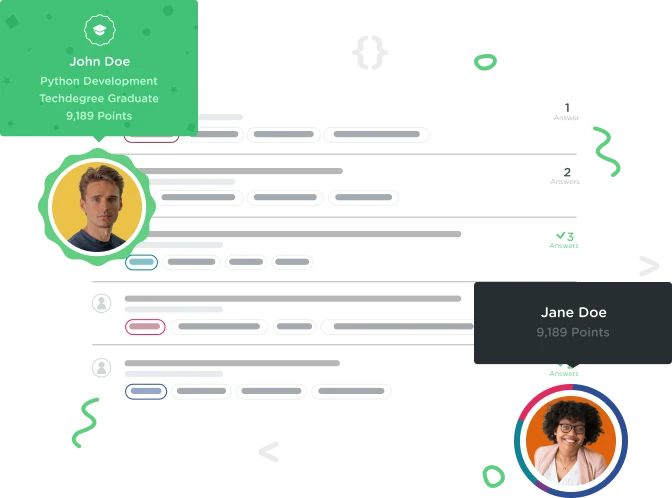

Ali Ahmed
53 PointsHow to change the degree to Celsius?
Hi I just want to know in the "Stormy App" How I can change the temperature in which file of the project? Thanks
1 Answer
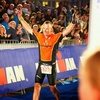
Steve Hunter
57,712 PointsHi Ali,
The temperature is held in the CurrentWeather
class instance called currentWeather
. There's a getter method that returns the value provided by the JSON data. At the moment, that's in Fahrenheit; you want a conversion to Celsius, I assume?
At the moment, the method is:
public int getTemperature() {
return (int)Math.round(mTemperature);
}
So, you need to tinker with mTemperature
before it is returned. Converting from F to C involves subtracting 32, then multiplying the result by 5/9. (I think!!)
So, something like:
public int getTemperature() {
return (int)Math.round((mTemperature -32) * (5/9));
}
That might do the trick. I hope that helps and works!!
Steve.
ammonquackenbush
3,867 Pointsammonquackenbush
3,867 PointsGood idea.
Brendan Milton
9,050 PointsBrendan Milton
9,050 PointsHi Steve!
I read over your info on converting from F to C, Im just struggling with how to use the method in the current weather program? would it be used in conjunction with one of the following lines of code?
ie: "current.setTemperature(currently.getDouble("temperature"));" Towards the bottom?
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsHi Brendan Milton
I implemented the conversion in the class definition. So, inside the Class definition for, say,
Current
, I have:I have the same in the
Hour
class. Within theDay
class, I applied the maths within thegetTemperatureMax()
method.Yes, you can use fewer lines of code just return the calculation, but I prefer the more verbose approach as it makes the calculation clearer, for me.
I hope that makes sense.
Steve.