Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial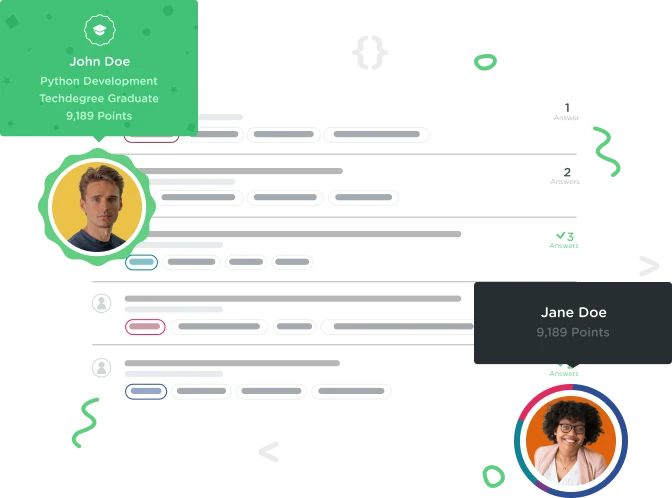
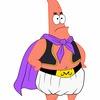
<noob />
17,062 PointsHow to check if a the user entered a valid words from the word? examples inside, i will appriciate ur help
hi, craig said in the end of the video that we should add a way to block the users from, entering a invalid words from the word. I tried to accomplish this task but it dosent seem to work perfectly in the script for example let's say the word is "treehouse", if i try to enter the word "momo" i get the "try again messege", but if i try to enter the word "top" which is a valid word i also get the "try again messege". if i try for exmaple to enter the word "tree" or "house" i pass the check. after i googled for few min i found out that this method only work for whole words like "tree" and not like "top" which is a word that a user as thought of i will be happy to know if there is anyway to accomplish this task that will work for both sides, thanks!
def prompt_for_words(challange):
guesses = set()
print("What words can u find in the word {}".format(challange))
print("Enter Q to quit")
while True:
guess = input("{} words > . ".format(len(guesses)))
if guess.upper() == "Q":
break
elif guess.lower() not in challange_word:
print("{} not contains the words in the word {}, Try again..".format(guess, challange_word))
else:
guesses.add(guess.lower())
return guesses
3 Answers
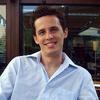
Pedro Cabral
33,586 Points"Top" is a word. And the letters "T" and "o" are present in the word "Treehouse" but the "p" isn't so that's why it turns out to be invalid. I think what he meant was that if you pick a word suck as "eerh" it will be valid because it's present in "Treehouse" but it should be invalid because it's not a real world. One check that you could do is to build a list of possible word combinations before hand and then make two checks:
- Are all the letters of the word the user submitted in the master keyword? Good, it's a candidate.
- Is the word the user submitted in the list of approved words? Good, it's a "real" word.
Come to think of it, and the first check is not even necessary, because if the user submitted word it is in the list of accepted words, that should mean that the characters that are present there are also present in the "master" keyword because you built the list taking that into account.
With that being said, your code is still faulty, because at the moment you are checking for user_input in keyword instead of all_characters_of_user_input in keyword which will allow for the mutations/scrambling them up. (This is what you mentioned about whole words: "Tree" or "house").
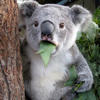
ursaminor
11,271 PointsThe way you have it now will error because you reference challange_word
in the function but it's not defined. I think you mean challange
. (BTW, the word is "challenge", not "challange".) Also, you need to lowercase the challenge word, otherwise looking for "tree" in "Treehouse" will return false.
elif guess.lower() not in challenge.lower():
To check if a word is in the English dictionary you can use a Python library like PyEnchant. https://stackoverflow.com/questions/3788870/how-to-check-if-a-word-is-an-english-word-with-python
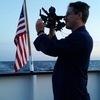
Seth Gorham
5,016 PointsHere's how I checked the guess against the challenge word:
def word_check(guess, challenge):
check_list = list(challenge)
for letter in list(guess):
if letter in check_list:
check_list.remove(letter)
else:
return False
return True
This doesn't examine case, but I covered that in the call to the function