Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial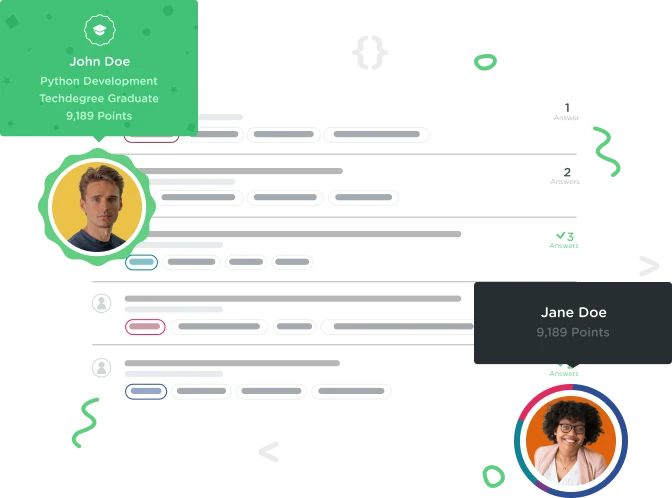
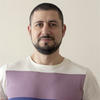
Daniel Petrov
3,495 PointsHow to check if an argument of a function is an integer?
Struggling with this challenge. It works without the first line: def add(arg1, arg2): but I think it must be there. Also not sure if I have to use input at all as the arguments may come from anywhere else. There is also some issue with "return(None)" I think
def add(arg1, arg2):
try:
arg1 = int(input("Enter your first digit: "))
arg2 = int(input("Enter your second digit: "))
except ValueError:
return(None)
else:
return(float(arg1) + float(arg2))
2 Answers
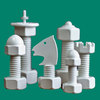
Steven Parker
231,275 PointsRemember that the arguments are being passed in to the function, you won't need to input them, and since this challenge is all about floats, checking for or converting to integers will not be used.
Just move your float-add-return line into the try block, and you won't need an else. So it would look like this:
def add(arg1, arg2):
try:
return(float(arg1) + float(arg2))
except ValueError:
return(None)
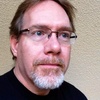
Chris Freeman
Treehouse Moderator 68,457 PointsThe short answer is
isinstance( <var>, int )
The longer answer is, for this challenge, you don't have to use the input()
function. The challenge grader will provide arguments when calling the function.
The other change is to convert to float
instead of int
For your code change:
Edit: change to float
from int
arg1 = int(input("Enter your first digit: "))
arg2 = int(input("Enter your second digit: "))
# to
arg1 = float(arg1)
arg2 = float(arg2)
If you wanted to test your code, you can call the function with arguments:
add(5, 2)
add("1", 5)
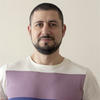
Daniel Petrov
3,495 PointsThanks Chris, that is something I tried as well before but for some reason, it did not work:
def add(arg1, arg2):
try:
arg1 = int(arg1)
arg2 = int(arg2)
except ValueError:
return(None)
else:
return(float(arg1) + float(arg2))
Is this what you mean?
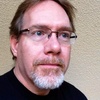
Chris Freeman
Treehouse Moderator 68,457 PointsI've corrected my answer to use float
. If int
were used then actual float
arguments would be converted to int
first then back to float
losing information.
Also, the conversion to float only needs to happen once making the
return` simple
Your whole code should be:
def add(arg1, arg2):
try:
arg1 = float(arg1)
arg2 = float(arg2)
except ValueError:
return None
else:
return arg1 + arg2
Note return
does not need the parens.
Daniel Petrov
3,495 PointsDaniel Petrov
3,495 PointsThanks Steven, the third part of the challenge is actually about making sure the arguments are integers.