Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial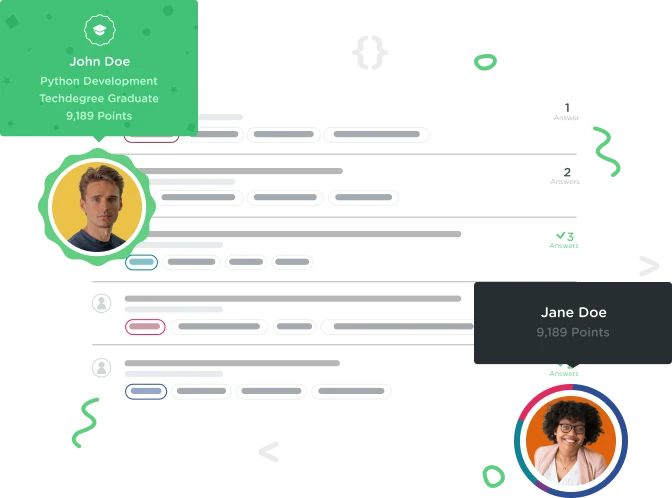
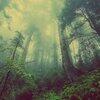
Jordan Howlett
4,377 PointsHow to combine functions. DRY practice.
How could I make these functions into a more DRY bit of code? The variables are image sources.
function bigFunction() {
document.getElementById("item-one").src = bigBirds;
document.getElementById("item-two").src = rhea;
document.getElementById("item-three").src = ralle;
document.getElementById("item-four").src = vulture;
}
// Second function, little birds
function littleFunction() {
document.getElementById("item-one").src = chickChasco;
document.getElementById("item-two").src = kingFisher;
document.getElementById("item-three").src = scalyMunia;
document.getElementById("item-four").src = tit;
}
// Third function, cool birds
function coolFunction() {
document.getElementById("item-one").src = coolBirds;
document.getElementById("item-two").src = erzglanz;
document.getElementById("item-three").src = macaw;
document.getElementById("item-four").src = yeater;
}
// Fourth function, weird birds
function weirdFunction() {
document.getElementById("item-one").src = groundHornbill;
document.getElementById("item-two").src = toucan;
document.getElementById("item-three").src = pompadour;
document.getElementById("item-four").src = silveryHornbill;
}
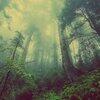
Jordan Howlett
4,377 PointsVery nice, Im transitioning from PHP to JavaScript so I was wondering if there were things like Getters/Setters that could be used to accomplish the same task in fewer lines of code.
1 Answer

Toluwalase Akintoye
Full Stack JavaScript Techdegree Graduate 22,958 Points//bird array
const firstArray = ['bigBirds', 'rhea', 'ralle', 'vulture'];
const secondArray = ['chickChasco', 'kingFisher', 'scalyMunia', 'tit'];
const thirdArray = ['coolBirds', 'erzglanz', 'macaw', 'yeater'];
const fourthArray = ['groundHornbill', 'toucan', 'pompadour', 'silveryHornbill'];
//bird function
function bigFunction(array) {
document.getElementById("item-one").src = array[0];
document.getElementById("item-two").src = array[1];
document.getElementById("item-three").src = array[2];
document.getElementById("item-four").src = array[3];
}
bigFunction(firstAarray);
//little birds
bigFunction(secondArray);
//cool birds
bigFunction(thirdArray);
//wierd birds
bigFunction(fourthArray);
Gergely Bocz
14,244 PointsGergely Bocz
14,244 PointsHi Jordan!
First of all, there are 4 functions, that essentially do the same: bigfunction(), littleFunction(), coolFunction() and weirdFunction() all change the value of src of each item. I believe they exist, because at different times you want to change the src to different values, but you can do that by giving the function parameters, and using those parameters to change the src values instead of the currently used variables. It would look like this:
If you want to also change the items, you can give them as parameters to the function aswell:
Now the problem with this, is that it really isn't that readable and it also forces you to change 4 elements all at once. In practice i would make it select and change only 1 element, so the function declaration would look something like this:
and call it as many times as needed, like so: