Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial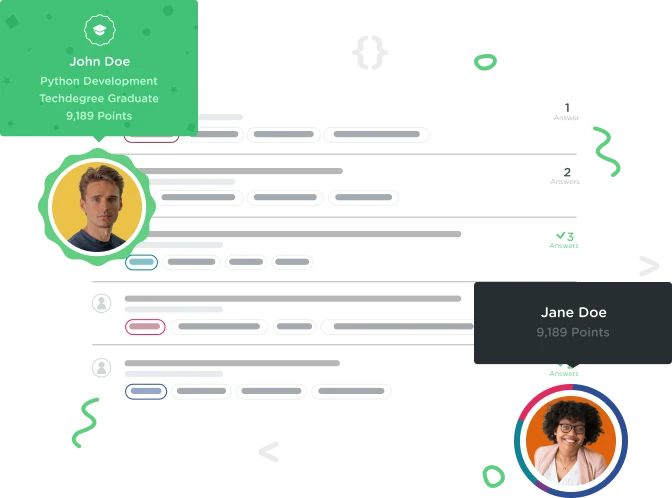

lettereyemedia
5,725 PointsHow to combine Javascript instead of repeating
I am looking to combine JS here to not repeat myself but my skills are not there yet and need some help, anything is appreciated!
$(function() {
<?php if ($showTeamData) {?>
/** Personal Total Graph **/
var $personalLoader = $("#PersonalTotal").percentageLoader({
width: 50,
height: 50,
controllable : false,
progress : 0.5,
value : '<?php echo $personalGoal;?>',
heading: '', symbal:'$',
color: 'black',
size: 'small',
onProgressUpdate : function(val) {
$personalLoader.setValue(Math.round(val * 100.0));
}
});
var personalLoaderRunning = false;
$(document).ready(function() {
if (personalLoaderRunning) {
return;
}
personalLoaderRunning = true;
var startZero = 0;
var goalDollars = <?php echo $personalGoal;?>;
var progressDollars = <?php echo $personalTotal;?>;
$personalLoader.setProgress(0);
$personalLoader.setValue('');
var i = 1;
if (progressDollars<10) {
i = 1;
} else if (progressDollars>=10 && progressDollars<50) {
i = 2;
} else if (progressDollars>=50 && progressDollars<100) {
i = 5;
} else if (progressDollars>=100 && progressDollars<1000) {
i = 10;
} else if (progressDollars>=1000) {
i = 50;
}
var animateFunc = function() {
startZero = startZero + i;
$personalLoader.setProgress(startZero / goalDollars);
$personalLoader.setValue('');
if (startZero < progressDollars) {
setTimeout(animateFunc, 25);
} else {
$personalLoader.setProgress(progressDollars / goalDollars);
personalLoaderRunning = false;
}
}
setTimeout(animateFunc, 25);
});
/** Team Members **/
var $memberLoader = $("#TeamMembers").percentageLoader({
width: 50,
height: 50,
controllable : false,
progress : 0.5,
value : '<?php echo $teamHeadcountGoal;?>',
heading: '',
color:'black',
size: 'small',
onProgressUpdate : function(val) {
$memberLoader.setValue(Math.round(val * 100.0));
}});
var memberLoaderRunning = false;
$(document).ready(function() {
if (memberLoaderRunning) {
return;
}
memberLoaderRunning = true;
var kb = 0;
var goalDollars = <?php echo $teamHeadcountGoal;?>;
var progressDollars = <?php echo $teamHeadcount;?>;
$memberLoader.setProgress(0);
$memberLoader.setValue('');
var i =1;
if (progressDollars<10) {
i = 1;
} else if (progressDollars>=10 && progressDollars<50) {
i = 2;
} else if (progressDollars>=50 && progressDollars<100) {
i = 5;
} else if (progressDollars>=100 && progressDollars<1000) {
i = 10;
} else if (progressDollars>=1000) {
i = 50;
}
var animateFunc = function() {
kb = kb +i;
$memberLoader.setProgress(kb / goalDollars);
$memberLoader.setValue('');
if (kb < progressDollars) {
setTimeout(animateFunc, 25);
} else {
$memberLoader.setProgress(progressDollars / goalDollars);
memberLoaderRunning = false;
}
}
setTimeout(animateFunc, 25);
});
/** Team Total **/
var $teamLoader = $("#TeamTotal").percentageLoader({
width: 160,
height: 160,
controllable : false,
progress : 0.5,
value : '<?php echo $teamGoal;?>',
heading:'Team Total', symbal:'$', color:'blue', onProgressUpdate : function(val) {
$teamLoader.setValue(Math.round(val * 100.0));
}});
var teamLoaderRunning = false;
$(document).ready(function() {
if (teamLoaderRunning) {
return;
}
teamLoaderRunning = true;
var kb = 0;
var goalDollars = <?php echo $teamGoal;?>;
var progressDollars = <?php echo $teamTotal;?>;
$teamLoader.setProgress(0);
$teamLoader.setValue('Goal: $'+goalDollars);
var i =1;
if (progressDollars<10) {
i = 1;
} else if (progressDollars>=10 && progressDollars<50) {
i = 2;
} else if (progressDollars>=50 && progressDollars<100) {
i = 5;
} else if (progressDollars>=100 && progressDollars<1000) {
i = 10;
} else if (progressDollars>=1000) {
i = 50;
}
var animateFunc = function() {
kb = kb +i;
$teamLoader.setProgress(kb / goalDollars);
$teamLoader.setValue('Goal: $'+goalDollars);
if (kb < progressDollars) {
setTimeout(animateFunc, 25);
} else {
$teamLoader.setProgress(progressDollars / goalDollars);
teamLoaderRunning = false;
}
}
setTimeout(animateFunc, 25);
});
<?php } else { ?>
/*Personal Total*/
var $teamLoader = $("#TeamTotal").percentageLoader({width: 200, height: 200, controllable : false, progress : 0.5,
value : '<?php echo $personalGoal;?>', heading:'Personal Total', symbal:'$', color:'blue', onProgressUpdate : function(val) {
$teamLoader.setValue(Math.round(val * 100.0));
}});
var teamLoaderRunning = false;
$(document).ready(function() {
if (teamLoaderRunning) {
return;
}
teamLoaderRunning = true;
var kb = 0;
var goalDollars = <?php echo $personalGoal;?>;
var progressDollars = <?php echo $personalTotal;?>;
$teamLoader.setProgress(0);
$teamLoader.setValue('Goal: $'+goalDollars);
var i =1;
if (progressDollars<10) {
i = 1;
} else if (progressDollars>=10 && progressDollars<50) {
i = 2;
} else if (progressDollars>=50 && progressDollars<100) {
i = 5;
} else if (progressDollars>=100 && progressDollars<1000) {
i = 10;
} else if (progressDollars>=1000) {
i = 50;
}
var animateFunc = function() {
kb = kb +i;
$teamLoader.setProgress(kb / goalDollars);
$teamLoader.setValue('Goal: $'+goalDollars);
if (kb < progressDollars) {
setTimeout(animateFunc, 25);
} else {
$teamLoader.setProgress(progressDollars / goalDollars);
teamLoaderRunning = false;
}
}
setTimeout(animateFunc, 25);
});
<?php } ?>
});
1 Answer
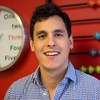
Nick Ocampo
15,661 PointsI'm not exactly sure what you mean by combining JS, but I recommend splitting this function (it's way too large) into smaller, more modular functions. Each function should perform one specific task, you can make a function that contains other functions inside of it too. It will make your code much more readable and easier to manage.