Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial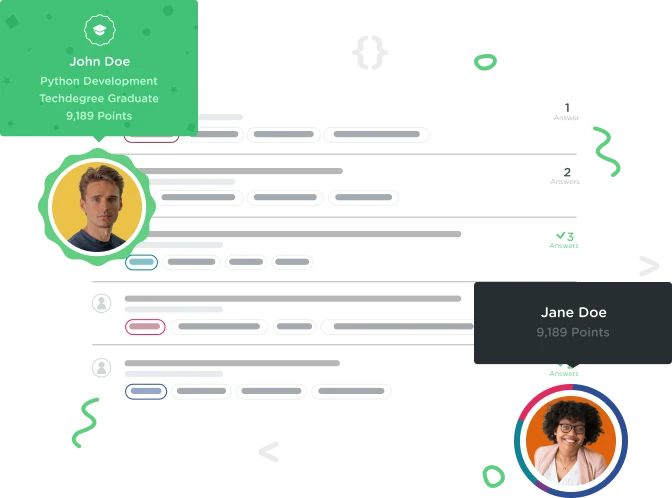
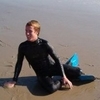
Jeremy Frimond
14,470 PointsHOW TO: Conditional Class Addition Using URL Bar
I have an unordered list with <a> lags that link to various id's on the same page. I am trying to add a class to the links when they are active.
What I want to do is write an IF statement to evaluate which link Im on. if the link is active then echo a command. What I do not know is what to put into the if statement to evalute. I imagine it will be the url because that is where the selected div is reflected?
here is my code
<div class="credentialContainer grid6">
<ul class="grid6">
<li><a id="one" href="#education" class="<?php if(??????){ echo "active"; } ?>">Education</a></li>
<li><a id="two" href="#designations" class="<?php if(??????){ echo "active"; } ?>">Designations</a></li>
<li><a id="three" href="#stateLicenses" class="<?php if(??????){ echo "active"; } ?>">State Licenses</a></li>
<li><a id="four" href="#affiliations" class="<?php if(??????){ echo "active"; } ?>">Affiliations</a></li>
</ul>
<div id="education" class="box">
<p>This is the education box</p>
</div>
<div id="designations" class="box">
<p>This is the designations box</p>
</div>
<div id="stateLicenses" class="box">
<p>This is the license Box</p>
</div>
<div id="affiliations" class="box">
<p>This is the affiliations box</p>
</div>
</div>
2 Answers
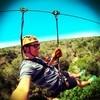
thomascawthorn
22,986 PointsThe way you've structured your code, you're better off using jquery (javascript) to grab the hash from the url.
You can check the current hash (otherwise known as the fragment) in the url using this javascript:
if (window.location.hash) {
// Fragment exists
} else {
// Fragment doesn't exist
}
window.location.hash
will also be the value of the hash.
You might add a class to the anchor tags and write an onclick listener:
class:
<li><a id="one" href="#education" class="navigation-link">
$('.navigation-link').click(function(e) {
e.preventDefault();
$('.navigation-link').removeClass('selected');
$(this).addClass('selected');
});
you might add something to your document ready function to auto-select the link the same way.
$('document').ready(function() {
if (window.location.hash) {
activateNavigationLink(window.location.hash);
}
});
function activateNavigationLink(hash) {
$('.navigation-link[href="#'+ hash +'"]').addClass("active");
}
You could even combine all of that into one super, less repetitive function and be much better than I am.
p.s. I haven't tested any of this, so might not be correct! :p
If you're looking to use php for this, you're better off not using a fragment and instead using a query string and pulling out and comparing the $_GET['some-variable'] to the link. You might have this url to access the page:
<?php
if ($_GET['active'] == 'education') { echo 'selected'; } ?>
Hope this point you in the right direction.
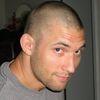
Jeremy Germenis
29,854 PointsWhat you are trying to do would be better achieved in jQuery ( mouseover ) but if you are trying to do this in php, I would...
1) include a get variable in the url like href="?section=education#education"
2) write an if statement to check if the section variable is present and what the value is
3) then execute based on the value