Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial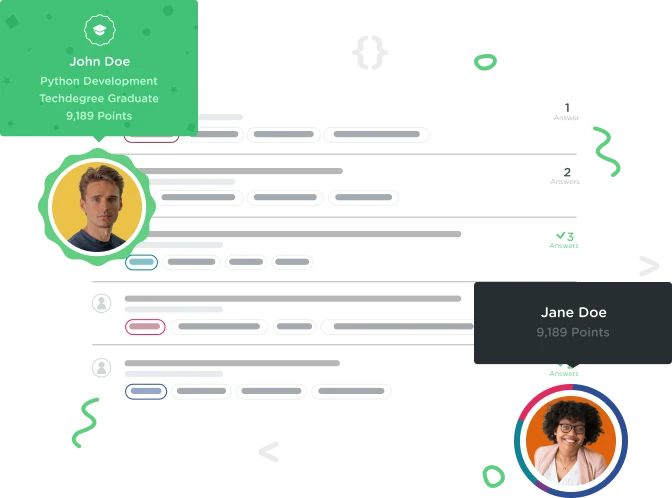

jake jake
Courses Plus Student 812 Pointshow to convert a number integer into a percentage PLEASE HELP
hi I'm trying to build an app which involves adding, subtracting and dividing numbers and I already wrote out the code and it works but I want to convert the answer into a percentage. how do I do that? thanks
3 Answers

Daniel Hartin
18,106 PointsHi Jake
Sorry I've not answered sooner, I have been busy for a few days. Is it right that you wish to display thesum in textbox t1 and not total which is your string variable.
Secondly all of your variables are set to the data type integer which is fine if that's what you want however be wary because if the number gets very small at some point the data type integer doesn't support decimal numbers and will round down to zero which I think may be happening here, why not try setting the data type as a double which do support decimal numbers.
and lastly in order to divide 2 integers using the / operator you must specifically cast one of the values as a double in order to return a decimal value otherwise the value will revert to a whole number.
Check out the code below for a sample class I created and successfully tested at my end here, if you get any problems feel free to post back here
package uk.co.trentbarton.myapplication;
import android.app.Activity;
import android.os.Bundle;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
import java.text.DecimalFormat;
public class MainActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
final EditText e1 = (EditText) findViewById(R.id.editText1);
final EditText e2 = (EditText) findViewById(R.id.editText2);
final EditText e3 = (EditText) findViewById(R.id.editText3);
final EditText e4 = (EditText) findViewById(R.id.editText4);
final EditText e5 = (EditText) findViewById(R.id.editText5);
final EditText e6 = (EditText) findViewById(R.id.editText6);
final EditText e7 = (EditText) findViewById(R.id.editText7);
final EditText e8 = (EditText) findViewById(R.id.editText8);
final EditText e9 = (EditText) findViewById(R.id.editText9);
final EditText e10 = (EditText) findViewById(R.id.editText10);
final TextView t1 = (TextView)findViewById(R.id.textView);
Button button = (Button) findViewById(R.id.button);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
int n1;
int n2;
int n3;
int n4;
int n5;
int n6;
int n7;
int n8;
int n9;
int n10;
double thesum;
double x;
String total;
n1 = Integer.parseInt(e1.getText().toString());
n2 = Integer.parseInt(e2.getText().toString());
n3 = Integer.parseInt(e3.getText().toString());
n4 = Integer.parseInt(e4.getText().toString());
n5 = Integer.parseInt(e5.getText().toString());
n6 = Integer.parseInt(e6.getText().toString());
n7 = Integer.parseInt(e7.getText().toString());
n8 = Integer.parseInt(e8.getText().toString());
n9 = Integer.parseInt(e9.getText().toString());
n10 = Integer.parseInt(e10.getText().toString());
try {
x = ((n1 + n2 + n3 + n4 + n5 + n6 + n7) / (double) (n8 + n9 + n10));
}catch(Exception e){
x = 0.00;
}
thesum = (x * 10);
DecimalFormat form = new DecimalFormat("0.00");
total = form.format(thesum) + "%";
t1.setText(total);
}
});
Thanks Daniel
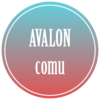
AVALONcomu Profile
36,146 PointsYou should try this: IN_PERS = CURRENT_NUMBER/SUM_OF_NUMBERS *100

jake jake
Courses Plus Student 812 Pointsit doesn't accept that code and I cant find a reference for it
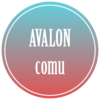
AVALONcomu Profile
36,146 Pointslet`s try again in details...
int answer = number / sum * 100;
where number is variable with answer numbe, and sum is variable of sum of all number or whatever you want
or if you need just output like that: 100% you can do that:
String variableName = integerVariable + "%";

jake jake
Courses Plus Student 812 Pointsthis is what I have right now but when I run the app the answer only comes out as 0. it wont make the number a decimal or convert it to a percentage
public void OnButtonClick (View v) {
int n1;
int n2;
int n3;
int n4;
int n5;
int n6;
int n7;
int n8;
int n9;
int n10;
int thesum;
int x;
String total;
EditText e1 = (EditText) findViewById(R.id.num1);
EditText e2 = (EditText) findViewById(R.id.num2);
EditText e3 = (EditText) findViewById(R.id.num3);
EditText e4 = (EditText) findViewById(R.id.num4);
EditText e5 = (EditText) findViewById(R.id.num5);
EditText e6 = (EditText) findViewById(R.id.num6);
EditText e7 = (EditText) findViewById(R.id.num7);
EditText e8 = (EditText) findViewById(R.id.num8);
EditText e9 = (EditText) findViewById(R.id.num9);
EditText e10 = (EditText) findViewById(R.id.num10);
TextView t1 = (TextView)findViewById(R.id.sum);
n1 = Integer.parseInt(e1.getText().toString());
n2 = Integer.parseInt(e2.getText().toString());
n3 = Integer.parseInt(e3.getText().toString());
n4 = Integer.parseInt(e4.getText().toString());
n5 = Integer.parseInt(e5.getText().toString());
n6 = Integer.parseInt(e6.getText().toString());
n7 = Integer.parseInt(e7.getText().toString());
n8 = Integer.parseInt(e8.getText().toString());
n9 = Integer.parseInt(e9.getText().toString());
n10 = Integer.parseInt(e10.getText().toString());
x = ((n1 - n2 - n3 - n4 - n5 - n6 - n7) / (n8 + n9 + n10));
thesum = (x/10 * 100);
total = thesum + "%";
t1.setText(Integer.toString(thesum));
jake jake
Courses Plus Student 812 Pointsjake jake
Courses Plus Student 812 Pointshey thanks man I appreciate the help I couldn't figure this out you really helped me out