Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial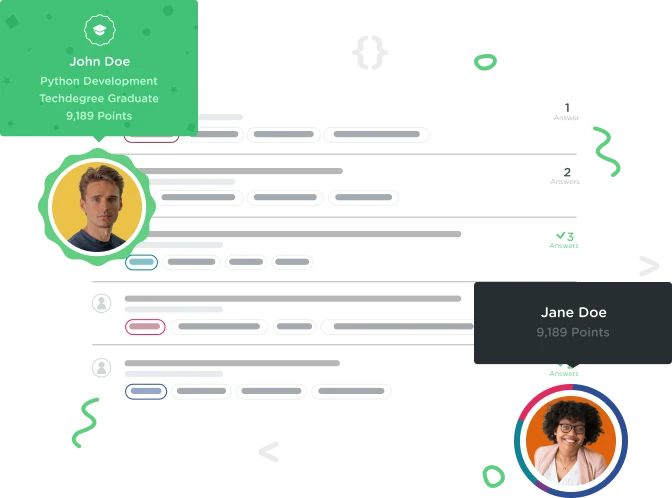

Xavier Walton
406 Pointshow to convert the string into int
def suggest(product_idea):
product_idea= int(product_idea)
if product_idea <3:
raise ValueError ("What is you doing?")
else:
return product_idea + "inator"
2 Answers

Cynthia Vera
Python Development Techdegree Student 1,838 PointsI think this is what you are looking for. Because you want to count the characters in the product_idea input, you gotta use len()
def suggest(product_idea):
if len(product_idea) <= 3:
raise ValueError("what is you doing?")
return product_idea + "inator"

Josh Stephens
13,529 PointsHi Xavier Walton I am not 100% what you are wanting to do with your code so I took a best guess at what you are wanting to do. I think the problem was that you were trying to concat a string and int together which is not possible without doing a cast of the int back to the string or doing interpolation like the following (I also rewrote it a little bit to be more verbose on the inputs and outputs)
In [4]: def suggest(product_idea: str) -> str:
...: product_idea = int(product_idea)
...: if product_idea < 3:
...: raise ValueError("What is you doing?")
...: return f"{product_idea}inator"
...:
In [5]: suggest(10)
Out[5]: '10inator'
In [6]: suggest(3)
Out[6]: '3inator'
In [7]: suggest(2)
---------------------------------------------------------------------------
ValueError Traceback (most recent call last)
<ipython-input-7-6cd087ab38b2> in <module>
----> 1 suggest(2)
<ipython-input-4-4f3c0c3ba03e> in suggest(product_idea)
2 product_idea = int(product_idea)
3 if product_idea < 3:
----> 4 raise ValueError("What is you doing?")
5 return f"{product_idea}inator"
6
ValueError: What is you doing?
In [8]:
Hopefully this helps but again I am not 100% sure on what you are trying to accomplish

Josh Stephens
13,529 PointsThinking on this a little more a better approach may be to do something like the below so that you can catch the different errors that might come through on your function. So if someone try to pass in an int it will still do your check on if it is greater less than 3 and if they pass in a string of 2 then it will still try and do your check and then at the end no matter if they passed in an int or a string it will still cast it
In [17]: def suggest(product_idea: str) -> str:
...: try:
...: if int(product_idea) < 3:
...: raise ValueError('What is you doing?')
...: return str(product_idea)+'inator'
...: except (ValueError, TypeError) as exc:
...: raise
...:
...:
In [18]: suggest(10)
Out[18]: '10inator'
In [19]: suggest(2)
---------------------------------------------------------------------------
ValueError Traceback (most recent call last)
<ipython-input-19-6cd087ab38b2> in <module>
----> 1 suggest(2)
<ipython-input-17-d82d16385372> in suggest(product_idea)
2 try:
3 if int(product_idea) < 3:
----> 4 raise ValueError('What is you doing?')
5 return str(product_idea)+'inator'
6 except (ValueError, TypeError) as exc:
ValueError: What is you doing?
In [20]: suggest(3)
Out[20]: '3inator'
In [21]: