Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial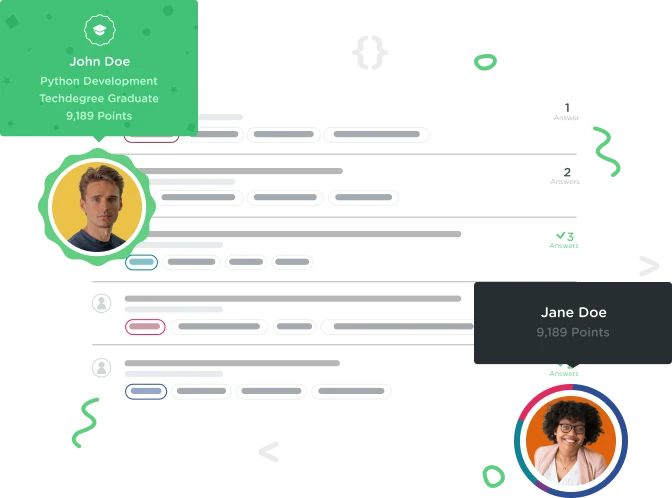

John Greenhoe
1,900 PointsHow to count a total number of items in an array
Hi,
I'm using wordpress as my backend and for each post I will have an array of cards, with each post having a different number of cards. I wanted to be able to count and display the total number of cards in that array on the post.
In the array some of the cards will needed to be counted twice as there are two of the same cards, for example one post may have 9 cards, but 6 of the 9 will have 2 copies of that card with the other 3 just having 1 so the total number of cards for that post will be 15.
The array I have the cards stored into is shown below.
<?php
$class_cards[]=array('card_count'=>$card_count,'has_term'=>$has_term,'card_img_class'=>$card_img_class,'card'=>$card,'card_title'=>$card_title,'card_mana'=>$card_mana,'card_slug'=>$card_slug);
?>
Each card has the variable $card_count which has either 1 or 2 next to it which is the number of copies of that card.
The loop I wrote to try to figure out the total number of cards is
<?php
$total_count_cards = count($class_cards);
foreach($class_cards as $key => $value)
{
if($value['card_count'] == 2 )
{
$total_count_cards++; // Adding another card if the card count is 2
}
}
echo $total_count_cards;
?>
Although this just shows that I have 9 cards instead of the 15, so it doesn't appear to be adding the extra cards for the cards that have 2 copies instead of one.
1 Answer
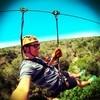
thomascawthorn
22,986 PointsSo this:
<?php
'card_count'=>$card_count
contains the total number of cards for a single $class_card?
Try:
<?php
$count = 0;
foreach($class_cards as $key => $value)
{
$count += $value['card_count'];
}
$count // should have total number
If the card count is an array, you could use php count() function to count all the items in an array.
John Greenhoe
1,900 PointsJohn Greenhoe
1,900 PointsThe
Contains either the number 1 or 2, as it is saying if that card in the array just has 1 copy or two, so I was trying to use that in the loop and if it contains 2 then add 1 additional card since using the php count function returns just 9 since there are 9 cards in the array, I need to look in the array at each card's card_count and if it contains a 2 then count that card twice.
thomascawthorn
22,986 Pointsthomascawthorn
22,986 PointsAnd if it's in there once, you need to count it once?
If so... I'm still convinced my suggested code should work! But maybe I've misunderstood the question ;)
John Greenhoe
1,900 PointsJohn Greenhoe
1,900 PointsYour suggestion returns 0 as the card count, to try to reword it better
Each post will have a set number of cards for this example lets say 9, they are all stored in the $class_cards array as seen in the first post. With each card it will have a $card_count that is either 1 or 2, this tells us if there are two copies of that card or just 1 copy of that card.
So for the original 9 cards 6 of them will have a count of 2, while 3 will have a count of 1 which would give us a total of 15 cards.
thomascawthorn
22,986 Pointsthomascawthorn
22,986 PointsLike this?
If so, both mine and your methods should work.. So my next suggestion would be to dump out one of these arrays before the loop - are the values as you expect? i.e. are they being set properly?