Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial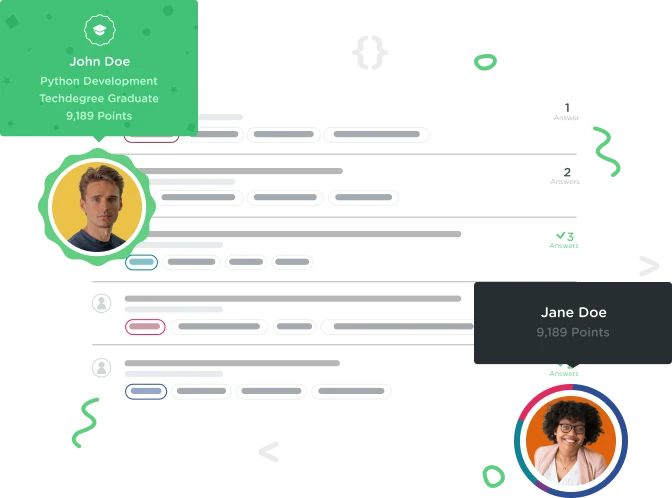

Ian Svoboda
16,639 PointsHow to count the number of times a specific character appears in a string?
I'm interested in how to pull the number of times a character appears in a string.
Example:
var exampleString = 'I will practice survival skills';
Desired output:
var result = 'L appears 5 times in the above string';
I tried to do some research on this but I haven't really found a solid method for doing so. Any help would be appreciated.
6 Answers

Michael Collins
433 PointsLet's try something a little simpler. Checkout javascripts match() function. Let's use that in combination with a regex. In this example, the regular expression is /d/gi. This means we are looking for occurrences of the letter "d". The "g" is a regex modifier. It means "global". So look for all occurrences of the letter "d". Without the "global" modifier, the regex would just stop on the first "d". The i is another regex modifier. It means "case insensitive".
var str = "This is the way I walk my dog";
var num_matches = str.match(/d/gi).length;
alert(num_matches); // This is number of times that "d" appears in the sentence
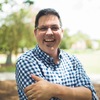
Dave McFarland
Treehouse TeacherYou could also make it case sensitive / or insensitive to search for appearances of letters that match both the upper and lowercase versions like this:
function letterCount(string, letter, caseSensitive) {
var count = 0;
if ( !caseSensitive) {
string = string.toUpperCase();
letter = letter.toUpperCase();
}
for (var i=0, l=string.length; i<string.length; i += 1) {
if (string[i] === letter) {
count += 1;
}
}
return count;
}
var exampleString = 'I will practice survival skills';
console.log(letterCount(exampleString, 'i', true)); // 4 (doesn't match uppercase I at beginning)
console.log(letterCount(exampleString, 'i', false)); 5 (matches both upper and lowercase i)

Ian Svoboda
16,639 PointsInteresting! I don't really need that sort of functionality, but I'll be studying your example just for learning purposes.
I appreciate the quick response here Dave! :)

Stone Preston
42,016 Pointsuse a function that loops through a string passed in as a parameter, and inside use a if statement to check if a character passed in as a parameter is equal to the index i of that string. if it is equal to it, increment a counter variable
//define your function
function characterCount(word, character) {
//initialize the counter to 0
var count = 0;
//loop through the word
for (i = 0; i < word.length; i++) {
//if the character in the word is equal to the character passed in as a parameter increment count
if (word[i] == character) {
count++;
}
}
//return the sentence.
return "The letter " + character + " appears " + count + " times in the word " + word ;
}
then call it like this:
characterCount("asdf asdf asdf", 'a');

Ian Svoboda
16,639 PointsStone, I think this is quite possibly the thing I'm looking for! The concept was just about what I had pictured, I just was having trouble putting it together (still learning some JS)—just started Dave's AJAX basics course (@Dave, you're a fantastic teacher by the way).
I wonder if you have an idea for the next part, which I admit I thought would be more straightforward.
I have an array of 27 total characters (essentially the alphabet and an underscore say) and I wanted to run this function for each item in that array.
Think of it like this: Check how many times every letter in the whole alphabet appears inside a given string.
Here is what I have so far:
var stringBase = ['a','b','c','d','e','f','g','h','i','j','k','l','m','n','o','p','q','r','s','t','u','v','w','x','y','z','_']
for (var i = 0; i < stringBase.length; i++) {
var currentChar = stringBase[i];
characterCount(theText, currentChar);
}
The trouble is that the function doesn't run for each item in the array as expected. In the console, it just runs the first item then doesn't output any further. I changed your original function just to include a console.log vs a return statement (either didn't seem to make much of a difference) and I tested that for loop to output the currentChar variable to the console to make sure it worked.
When I did this, the for loop output each element in the stringBase array one at a time, which is what I expected. I'm curious as to why this wouldn't work the same with the above example? I was thinking there may have been something in the function that caused the for loop to stop early, but I can't really see anything that would cause that sort of behavior.
You guys have all been fantastic and I sincerely appreciate your assistance. Any clarification here would be much appreciated.
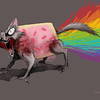
Tomas Dvorak
10,010 PointsTry this:
var exampleString = 'I will practice survival skills';
var count = exampleString.split('L').length-1;
var result = 'L appears' + count + 'times in the above string';
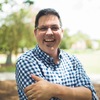
Dave McFarland
Treehouse TeacherHi Ian Svoboda
There are probably a lot of ways to do what you're after, but I'm thinking of creating an object that holds the count for each letter in your array. When it's done, you'll end up with a JavaScript object like this:
{
a : 1,
b : 5,
c : 4 // .... and so on and so on.
var exampleString = 'i will practice survival skills';
var stringBase = ['a','b','c','d','e','f','g','h','i','j','k','l','m','n','o','p','q','r','s','t','u','v','w','x','y','z','_'];
var countObject = {} ;
function characterCount(word, character) {
var count = 0;
for (var i = 0; i < word.length; i++) {
if (word[i] === character) {
count++;
}
}
return count;
}
for (var i = 0, l = stringBase.length; i < l; i++) {
var currentChar = stringBase[i];
countObject[currentChar] = characterCount(exampleString, currentChar);
}
console.log(countObject);

Ian Svoboda
16,639 PointsThanks for the quick reply! So word argument would just be the variable or string that I want to use then? I think this should work just fine.
Dave McFarland
Treehouse TeacherDave McFarland
Treehouse TeacherI like the way you think Michael Collins
Here's that as a function:
Michael Collins
433 PointsMichael Collins
433 PointsHey Dave ... it's been a long time. Fancy meeting you here! :)
Dave McFarland
Treehouse TeacherDave McFarland
Treehouse TeacherBuddy?
Michael Collins
433 PointsMichael Collins
433 PointsYep. That be me. Waz up?