Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial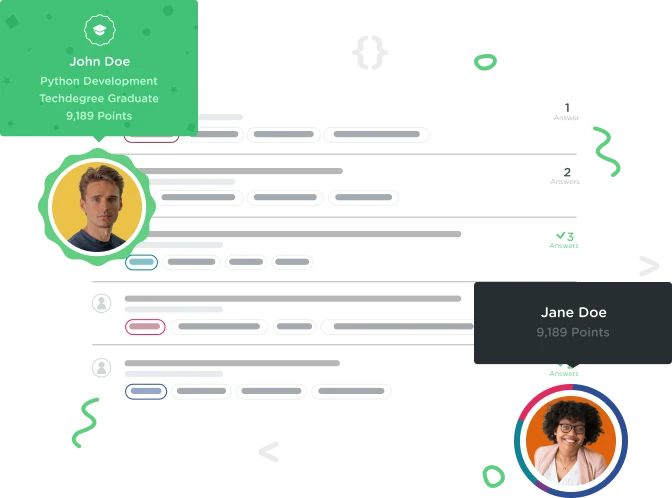

Gustavo Guzmán
4,213 PointsHow to create a function with arguments that work on the timedelta function???
I really tried, but can't find a solution that works: the aim is to create a function that takes 'minutes', 'seconds', 'days', 'years', along with an interger as arguments, and so these can be passed on to a datetime.timedelta function/method. However, timedelta is very specific about how to pass the arguments to it, e.g. 'days=4', so other arg names dont work.
I found on the internet an alternative, which would be creating a dict with the keys and values, and passing that on to the timedelta, but that still wouldn't work for this challenge!
How do you solve it?
import datetime
starter = datetime.datetime(2015, 10, 21, 16, 29)
# Remember, you can't set "years" on a timedelta!
# Consider a year to be 365 days.
## Example
# time_machine(5, "minutes") => datetime(2015, 10, 21, 16, 34)
def time_machine(num, duration):
if duration == 'years':
travel = (datetime.timedelta(days = 365)) * num
travel = datetime.timedelta(duration = num)
return starter + travel
2 Answers
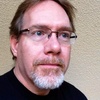
Chris Freeman
Treehouse Moderator 68,457 PointsHey Gustavo Guzmán, you’re on the right track.
Two errors to fix:
- the second assignment to
travel
should be in anelse:
block - the value of
duration
needs to be used as the keyword argument. As it is, the string “duration” is being used as the keyword.
One method to resolve this would be to use many if/elif statements to determine the value of duration
and set travel
accordingly.
A trick to accomplish this in one statement is to creat a dict the unpack it as a key/value pair:
datetime.timedelta(**{duration: num})
will create a dict of {“value_of_duration”: num}
, then by using **{}
this key/value pair will be unrolled just like **kwargs
Edit: adding another variable to dict option:
>>> dur = "days"
>>> value = 4
>>> dict([(dur, value)])
{‘month’: 4}
>>> datetime.timedelta(**dict([(dur, value)]))
datetime.timedelta(4)
Post back if you need more help. Good luck!!!

Gustavo Guzmán
4,213 PointsThank you very much! I've managed to get it using the many if/elifs. I didn't think it was very Pythonic, but it worked. I will definitely try the dictionary option, though, because I need to practice that.