Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial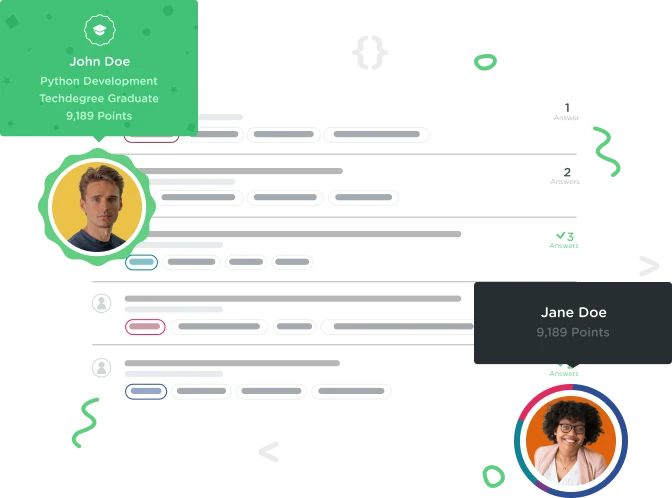
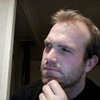
Marty Hitchcock
13,108 PointsHow to create a global variable inside a function
How do I set a global variable inside of a function? In the Treehouse video said it is bad practice to just drop the 'var' and put 'something = somethingelse', but it didn't offer an alternative.
Background: When the page loads the user is logged in with JS, so in order to access the current user I need to wait for that to happen. Once that has happened I would like to define some variables which would have data from the user. I have created a setInterval function to fire once the user is indentified, but I would like to use the variables defined inside the function outside of that function also.
3 Answers

Scott Vrable
5,248 PointsIf I'm understanding you correctly, there's a couple different ways you could do this.
For one, you can declare the variable(s) in the global space (preferably in a closure) but not assign it a value until you've retrieved that information from the user.
Example:
(function(){
var infoINeed;
function thingToDo() {
infoINeed = "whatever";
}
thingToDo();
// then you can use it down here, too
function anotherThing(infoINeed) {
// do something with infoINeed value...
}
})();
Another thing you could do would be to return an object upon getting the user information then pulling the content out of that object as needed.
function thingToDo() {
var infoINeed = "whatever";
return {
thing1: infoINeed,
thing2: "something else"
};
}
// assign the executed function to a variable for easy use
var obj = thingToDo();
// Then, later, you can get that information, this way:
obj.thing1;
Does that make sense?

Scott Vrable
5,248 PointsI had a mistake in the second version. I corrected it above.

Hunter Cassidy
5,674 PointsYou could pass the "global" object into your IIFE (immediately invoked function expression).
;(function(window) {
var myFunc = function() {
console.log('in my func...');
window.myVar = 5;
};
console.log(window.myVar); // -> undefined
myFunc();
})(window);
console.log(myVar); // -> 5
Marty Hitchcock
13,108 PointsMarty Hitchcock
13,108 PointsYes it does, thanks for that!