Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial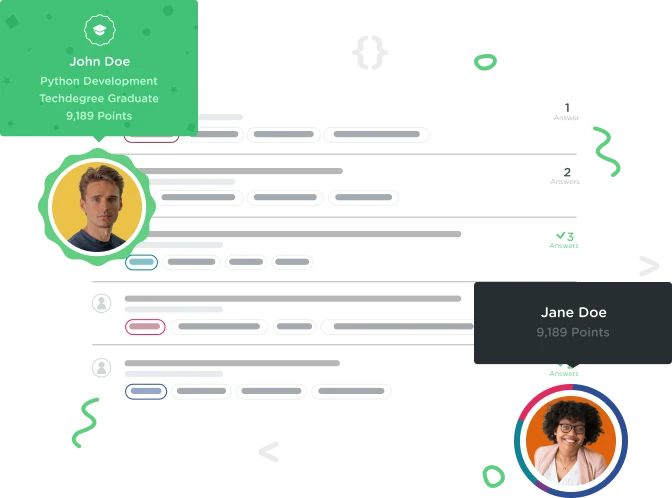

Trainer Workout
22,341 PointsHow to create an inner Handler class just like we did with GeolocationService.
// some methods omitted
public class MainActivity extends Activity {
Boolean isBound = false;
Messenger mServiceMessenger;
Messenger activityMessenger = new Messenger(new ActivityHandler(this));
ServiceConnection mServiceConnection = new ServiceConnection() {
@Override
public void onServiceConnected(ComponentName name, IBinder binder) {
isBound = true;
mServiceMessenger = new Messenger(binder);
} // onServiceConnected()
@Override
public void onServiceDisconnected(ComponentName name) {
isBound = false;
}
};
private class ActivityHandler extends Handler {
private MainActivity mainActivity;
public ActivityHandler(MainActivity mainActivity) {
this.mainActivity = mainActivity;
} // ActivityHandler()
} // ActivityHandler
} // MainActivity
// some methods omitted
public class GeolocationService extends Service {
int mLatitude;
int mLongitude;
Messenger mMessenger = new Messenger(new LocationHandler());
@Override
public IBinder onBind(Intent intent) {
return mMessenger.getBinder();
}
class LocationHandler extends Handler {
@Override
public void handleMessage(Message msg) {
mLatitude = getLatitude();
mLongitude = getLongitude();
} // handleMessage()
} // LocationHandler
} // GeolocationService
public class Difficulty {
public static Boolean HARDMODE = false;
}
3 Answers

Seth Kroger
56,414 PointsMuch like the GeoLocationService challenge, when the Handler is an inner class, you don't need to store a reference to the outer class object. This has to do with the way (non-static) inner classes work in general . Objects of inner classes can't exist outside of an object of the outer class and they automatically have access to the methods and member variables of the outer object. You don't need the reference to the MainActivity yourself because that reference is already embedded.

Urgent Nkala
8,772 Pointstry this: class mActivityHandler extends Handler{ @Override public void handlerMessage(Message msg) } } }

Gracious Chinyama
3,292 Pointsclass ActivityHandler extends Handler {
@Override
public void handleMessage(Message msg) {
mServiceMessenger = new Messenger(new ActivityHandler());
}
}
and it worked
Trainer Workout
22,341 PointsTrainer Workout
22,341 PointsLooks like I'm not learning from my mistakes :-). Thanks again.
Kevin Haube
12,265 PointsKevin Haube
12,265 PointsI'm attempting this without the reference to MainActivity and receiving the same error three years later.