Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial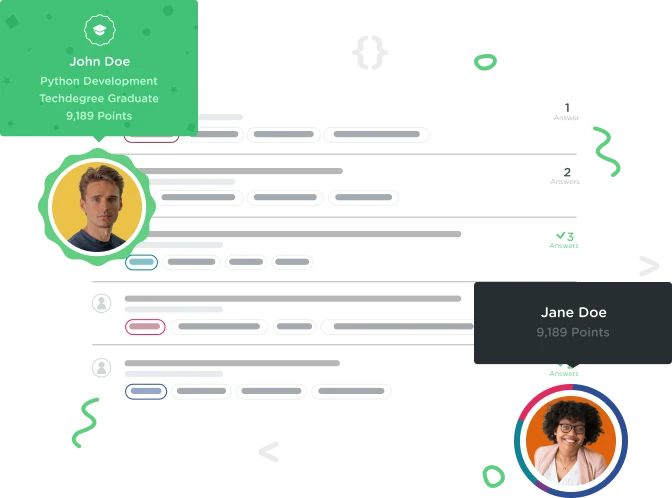

Diradj Bharosa
3,190 PointsHow to create an instance from a class - inheritance challenge
Hi guys, I do not understand what I did wrong here, the challenge was to create an instance of the class called round button that I created but it keeps giving me a 'bummer'.
The exercise: Create an instance of the class RoundButton and name the instance rounded.
This is what I have:
class Button { var width: Double var height: Double
init(width:Double, height:Double){ self.width = width self.height = height } }
class RoundButton: Button { var cornerRadius = 5.0 }
var rounded = RoundButton // This is what the challenge in question is about, the rest is background information.
Any help would be much appreciated, thanks!
2 Answers
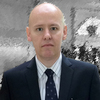
Nathan Tallack
22,159 PointsOk, first let's look at the code and how we would use it.
class Button {
var width: Double
var height: Double
init(width: Double, height: Double) { // This shows the paremeters we need to pass in to create it.
self.width = width
self.height = height
}
}
class RoundButton: Button { // No need for an init method here because we have no unset values.
var cornerRadius = 5.0 // This already has a default value so no need to pass it in when creating it.
}
var rounded = RoundButton(width: 20.0, height: 5.0) // We pass in the values to the init method.
So we have two takeaways here.
The first is we can see the properties (width and height) in the Button class have no default values so they need to be initialized. So we pass them in as paremeters when we make that object.
The second is that the subclass RoundButton has no properties that are defined without default values. So we can choose not to have a declared init method for this class and just use the one for the parent class.
The end result is your object (rounded) is initialized with the properties width=20.0, height=5.0 (these two we pass in) and cornerRadius=5.0 (this one is default).
I hope this helps. :) Keep up the great work!
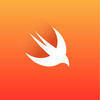
Steven Deutsch
21,046 PointsHey Diradj Bharosa,
You need to use parentheses and pass in values to the initializer method to create an instance of an object. Since your RoundButton class inherits from Button, also known as being a subclass of Button, you need to initialize the properties of the super class as well as it's own properties. In this situation, your RoundButton class has no properties that need to be initialized, because its only property, cornerRadius, is set to a default value. Therefore, you only need to initialize the values of the superclass to create a valid instance. See my code below:
class Button {
var width: Double
var height: Double
init(width:Double, height:Double){
self.width = width
self.height = height
}
}
class RoundButton: Button {
var cornerRadius = 5.0
}
let rounded = RoundButton(width: 5.0, height: 5.0)
Good Luck!

Diradj Bharosa
3,190 Pointsthanks for your explanation, I understand it better now.
Diradj Bharosa
3,190 PointsDiradj Bharosa
3,190 PointsThanks for the detailed explanation, this really helped.