Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial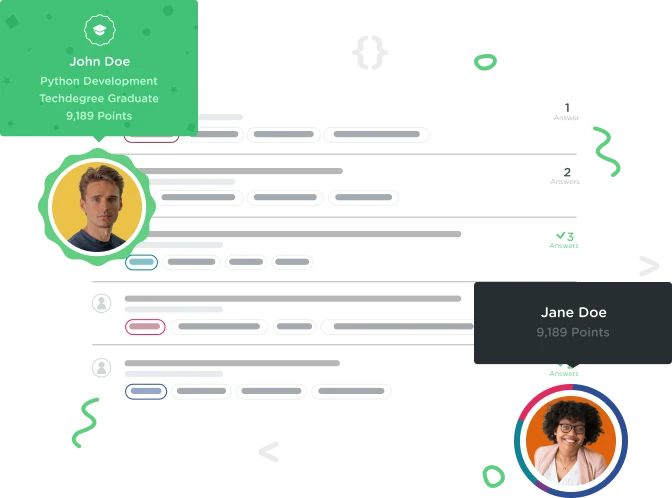
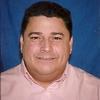
Jose Luis Ocaña
3,746 PointsHow to create life counter?
here is my snapshot https://w.trhou.se/2pb1iptbxf I am in the Wrapping It Up Introducing JavaScript. I am trying to create a Add a life counter. Instead of simply losing points when the player runs into a bottle of poison, why not start the game with 3 lives? Each time the player hits a bottle of poision they lose one life; at 0 lives the game is over. Wow I try other example from question in the chapter but not resolve. Can you help me?
2 Answers
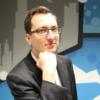
Ben Hobgood
1,791 PointsFirst, let's start with the simple stuff.
Let's start by creating the variables. We want to add three new variables "currentLife", "losingLife", and "lost". "currentLife" is where we set the initial number of lives as well as storing how many current lives the player has. "losingLife" is where we are going to store at what point when the player loses (most likely 0 is the best number for that)
Existing Code:
var won = false;
var currentScore = 0;
var winningScore = 100;
New Code:
var won = false;
var currentScore = 0;
var winningScore = 100;
var lost = false;
var currentLife = 3;
var losingLife = 0;
Now that we have stored our lives let's remove a life every time a player touches a poison by altering the existing code. It looks like you created a function to do this but you need to call it within the itemHandler function or, alternatively, if you see my example below you can simply remove lives. Finally we also want to check to see if "currentLife" is equal to "losingLife" and if so change lost to true to trigger the game over.
Existing Code:
function itemHandler(player, item) {
item.kill();
if (item.key === 'coin') {
currentScore = currentScore + 10;
} else if (item.key === 'star') {
currentScore = currentScore + 25;
}
if (currentScore === winningScore) {
createBadge();
}
}
New Code:
function itemHandler(player, item) {
item.kill();
if (item.key === 'coin') {
currentScore = currentScore + 10;
} else if (item.key === 'poison') {
currentLife = currentLife - 1;
} else if (item.key === 'star') {
currentScore = currentScore + 25;
}
if (currentLife === losingLife) {
lost = true;
}
if (currentScore === winningScore) {
createBadge();
}
}
Great! We have the functionality now but we can't see if it's doing anything. So let's add a counter. We don't have to figure this out from scratch, though! We already have a Score counter. We just have to copy how that's being created and edit it slightly. Note! If we copy it exactly it will overlap the score text so let's move it over to the right some.
Existing Code:
text = game.add.text(16, 16, "SCORE: " + currentScore, { font: "bold 24px Arial", fill: "white" });
New Code:
text = game.add.text(16, 16, "SCORE: " + currentScore, { font: "bold 24px Arial", fill: "white" });
life = game.add.text(600, 16, "LIVES: " + currentLife, { font: "bold 24px Arial", fill: "white" });
Well that's great we've got it on the board now but it's not updating the text.... Ah! Because we only updated the text within the "create" function. We need to also update it within the "update" function.
Existing Code:
text.text = "SCORE: " + currentScore;
New Code:
text.text = "SCORE: " + currentScore;
life.text = "LIFE: " + currentLife;
Finally, we need to trigger the GAME OVER Message and if we look a little bit further down the "update" function we can do what we've been doing so far and duplicating the "won" actions. Except we don't want users to be able to win after they lost so we're going to make this an if / else statement. This will prevent "winning" from happening as it will see lost first and will not continue further.
Exisiting Code:
if (won) {
winningMessage.text = "YOU WIN!!!";
}
New Code:
if (lost){
winningMessage.text = "YOU LOST!!!";
} else if (won) {
winningMessage.text = "YOU WIN!!!";
}
That should be everything! If you have any more questions let me know and if you'd like to see an example you can see a snapshot of my version here: https://w.trhou.se/1lloiizkrb

Mark Rogers
Python Development Techdegree Student 1,583 PointsI also found I had to change currentScore === winningScore to >= because of 25 value for star not matching winning score of 100. Game would not declare a winner.
// when the player collects an item on the screen function itemHandler(player, item) { item.kill(); if (item.key === 'coin') { currentScore = currentScore + 10; } else if (item.key === 'poison') { currentScore = currentScore - 25; } else if (item.key === 'star') { currentScore = currentScore + 25; } if (currentScore >= winningScore) { createBadge(); } }
Jose Luis Ocaña
3,746 PointsJose Luis Ocaña
3,746 PointsThanks Ben Hobgood. Great help. Thanks so much
Emanuel Rouse
1,985 PointsEmanuel Rouse
1,985 PointsFantastic comment explained everything beautifully. One additional question. I can see that the text variable is defined however the life variable is NOT defined yet it still works when executing this code :
life = game.add.text(600, 16, "LIVES: " + currentLife, { font: "bold 24px Arial", fill: "white" });
Any explanation as to why this works? Or am I missing something causing this to work?