Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial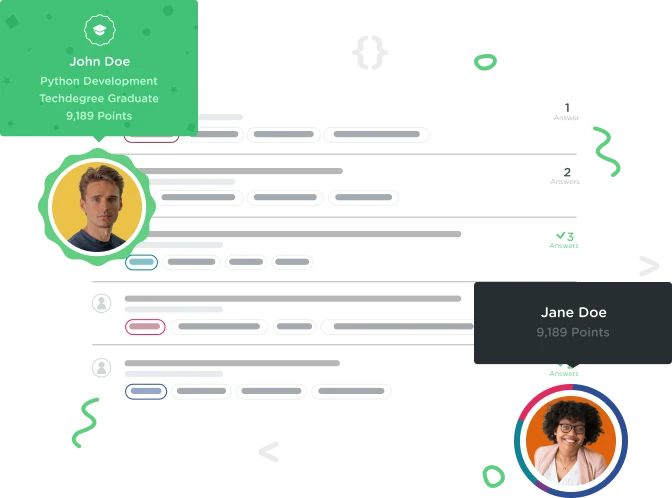
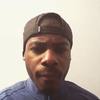
Tunde Adegoroye
20,597 PointsHow to create multidimensional array with key value pair JS
Hey everyone i'm currently learning more about javascript and wanted to know how to do something like this in PHP which is a multidimensional array with key pairs
$array = array(
"hilton" => array(
"name" => "hilton hotel"
"newton" => array(
"name" => "newton hotel"
) ;
But how would i do something like this in javascript this is what i have attempted and so far only got errors i'm using an object rather than array if i'm going about this wrong please tell me haha
var hotels = {
hilton = {
name: "hilton hotel"
}
newton = {
name: "newton hotel"
}
}
3 Answers

Daniel Newman
Courses Plus Student 10,715 PointsOh, I see! What you calling array is collection indeed. To access it like hotels["hilton"]["name"] you need such object:
var hotels = { "hilton": {"name": "hilton hotel" } };
So, the solution to keep this data you need is:
var hotels = {
"hilton": {"name": "hilton hotel" },
"newton": {"name", "newton hotel"}
};

Daniel Newman
Courses Plus Student 10,715 PointsWhy do you try to make an Object? In Object JS doing it other way(with ":" and ",")
var hotels = {
hilton : {
name: "hilton hotel"
},
newton : {
name: "newton hotel"
}
}
`
But if you need a multidimensional array do this way:
var hotels = [
[
"hilton",
["name", "hilton hotel"]
],
[
"newton",
["name", "newton hotel"]
]
];
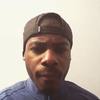
Tunde Adegoroye
20,597 PointsWould i just access the variables in the array like
hotels["hilton"]["name"];

Daniel Newman
Courses Plus Student 10,715 PointsNoup. See below how to keep data you need.
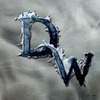
Hugo Paz
15,622 PointsHi Tunde,
Since an array is an object in javascript, would something like this suit you?
<script>
var multiarray = {
cat:{
name:'tobias',
legs:4
},
dog:{
name:'bobi',
legs:4
}
};
</script>
You can access each property either like this: multiarray.cat.name or if you prefer it to be like php: multiarray['cat']['legs']
Tunde Adegoroye
20,597 PointsTunde Adegoroye
20,597 PointsYour awesome haha sorry i thought it was called the same in js but thats exactly what i'm looking for are there any methods to add new items into the collection ?
Daniel Newman
Courses Plus Student 10,715 PointsDaniel Newman
Courses Plus Student 10,715 PointsTry to make it this way:
hotels["newone"] = {"name": "Whatever"};
Tunde Adegoroye
20,597 PointsTunde Adegoroye
20,597 PointsAwesome that works thanks for all your help mate :D
Daniel Newman
Courses Plus Student 10,715 PointsDaniel Newman
Courses Plus Student 10,715 PointsRegards!)
Andrew Chalkley
Treehouse Guest TeacherAndrew Chalkley
Treehouse Guest TeacherThese are commonly known as Object Literals in JavaScript but yes they are indeed associative arrays since the indexes are named (with a string) and not a numeric index.