Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial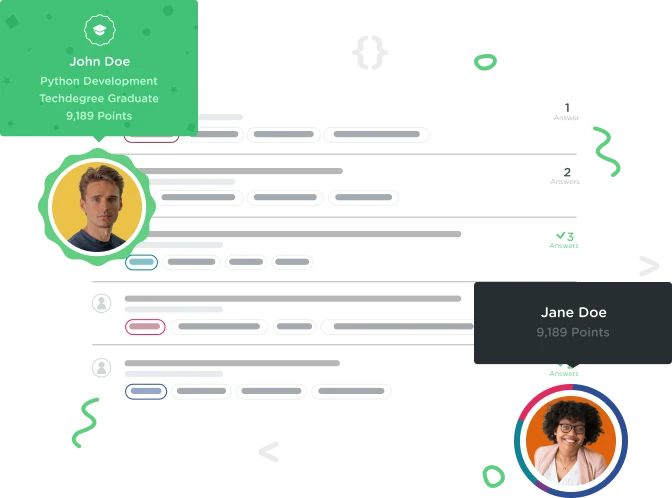

Igor Latychev
Courses Plus Student 7,310 PointsHow to create variables scoped to a function in JavaScript?
How does Let Var And Const all play a role in declaring variables in JavaScript? I find this concept hard to grasp for me personally so I just need that extra clarification to help me understand it better.
1 Answer

Clayton Perszyk
Treehouse Moderator 48,850 PointsHey Igor,
I'll try to explain each declaration.
var: unless var is declared in a function it will have global scope, otherwise it will be scoped to the function, i.e., any code outside of the function will not have access to it.
function foo(a, b) { // a and b will both be scoped locally to the function
var c = "Hello";
console.log(c); // this will log "Hello" to the console when foo is invoked
}
foo() // "Hello" logged to console
console.log(a); // this will cause an error since a is local to the function foo
console.log(b); // this will cause an error since b is local to the function foo
console.log(c); // this will cause an error since c is local to the function foo
var d = "World"; // this creates a global variable, which is available anywhere in your code, including functions.
// basically, from inside a function you can look out and but from without a function you cannot look in
// see below
function bar() {
console.log(d); // bar can access variables in global scope
}
console.log(d); // this will log world, since d is a global variable
// also, note that within any block (i.e. curly braces - {}), other than a function, var is scoped globally.
if (1 + 1 === 2) {
var e = "this is global";
console.log(e); // e is available here
}
console.log(e); // e is also available here
let: let is similar to var, except it creates scope within any block (i.e. anything between curly braces - {}).
if (1 + 1 === 2) {
let f = "this is scoped to this block";
console.log(f); // this will log the value of f
}
console.log(f); // this will throw an error, since f is available only within the conditional block
function baz() {
console.log(f); // this will also throw an error when the function is invoked
}
baz(); // f is only available within the conditional block
const: const is similar to let in that it creates block scope; however, where let can have values reassigned (i.e. updated), const can not change, i.e., it is constant.
if (1 + 1 === 2) {
const g = "this is local to this block and cannot be accessed elsewhere in the code, also it cannot be updated";
console.log(g); // this will log the value of g
}
console.log(g); // throws an error, since g is local to the conditional block
const h = "this is global, but constant"
const h = "can't assign new value to h"; // this will throw an error, since h can only hold the value it was assigned at
// declaration
One last thing about let vs var: a variable with a let declaration can only be declared once, whereas with var, it can be declared multiple times, but is not a good practice.
let myLetVariable = "all good";
myLetVariable = "still good"; // you can reassign to myLetVariable, but not redeclare
let myLetVariable = "no good" // this will throw an error, since myLetVariable was already declared
var myVarVariable = "all good"; // declare myVarVariable
myVarVariable = "still good"; // reassign to myVarVariable
var myVarVariable = "this will still be good"; // redeclare myVarVariable
Lastly, unless you have a good reason to use var, stick with let and const.
Hope this helps!