Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial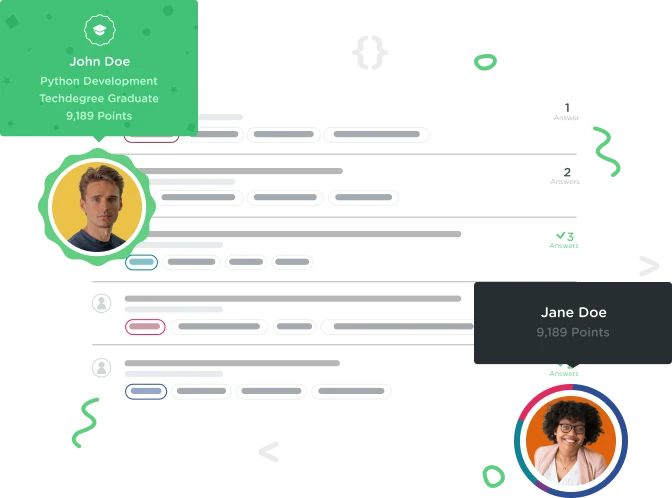

pogtynmsax
4,083 PointsHow to declare a function named coordinates with single parameter of type String, returns tuple of two Double values...?
how to declare a function named coordinates with single parameter of type String, returns tuple of two Double values, the return value does not have to be named. For example: If I use your function and pass in the String "Eiffel Tower" as an argument. I should get (48.8582, 2.2945) as the value. If a string is passed in that does not match the set above, return (0,0).
// Enter your code below
func coordinates (for location: String)-> (Double, Double) {
var lat = 0.0
var lon = 0.0
switch location {
case "Eiffel Tower":
lat = 48.8582
lon = 2.2945
case "Great Pyramids":
lat = 29.9792
lon = 31.1344
case "Sydney Opera House":
lat = 33.8587
lon = 151.2140
default:
lat = 0.0
lon = 0.0
}
return (lat, lon)
}
var locationOf = coordinates (for: "Eiffel Tower")
2 Answers

Alex Souto-Maior
iOS Development Techdegree Student 4,909 PointsHey Dawry,
You created two parameters of type Double. This challenge is asking for you to return a tuple of two Double values.
So you should create a parameter of type tuple to begin with.
Here's a suggestion of how the first few lines could start...
func coordinates(for location: String) -> (Double, Double) {
var locationCoordinates: (Double, Double) = (0, 0)
switch location {
case "Eiffel Tower": locationCoordinates = (48.8582, 2.2945)
etc...
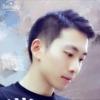
JUN MENG
10,249 Pointsfunc coordinates (for location: String) -> (Double, Double) {
var spot: (Double, Double) = (0, 0)
switch location {
case "Eiffel Tower": spot = (48.8582, 2.2945) case "Great Pyramid": spot = (29.9792, 31.1344) case "Sydney Opera House": spot = (33.8587, 151.2140) default: spot = (0.0, 0.0)
} return spot } var locationOF = coordinates (for: "Eiffel Tower")
Micah Doulos
17,663 PointsMicah Doulos
17,663 PointsAlex your comment is inconsistent with the directions.
"Declare a function named coordinates that takes a single parameter of type String, with an external name for, a local name of location, and returns a tuple containing two Double values (Note: You do not have to name the return values). For example, if I use your function and pass in the string "Eiffel Tower" as an argument, I should get (48.8582, 2.2945) as the value. If a string is passed in that doesnβt match the set above, return (0,0)"
Alex Souto-Maior
iOS Development Techdegree Student 4,909 PointsAlex Souto-Maior
iOS Development Techdegree Student 4,909 PointsHi Micah,
I don't understand what you see as an inconsistency.
This is the solution I used. It meets the requirements and passes the code challenge. If you have any questions feel free to reach out.
func coordinates(for location: String) -> (Double, Double) {
}
let location = coordinates(for: "Sydney Opera House")