Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial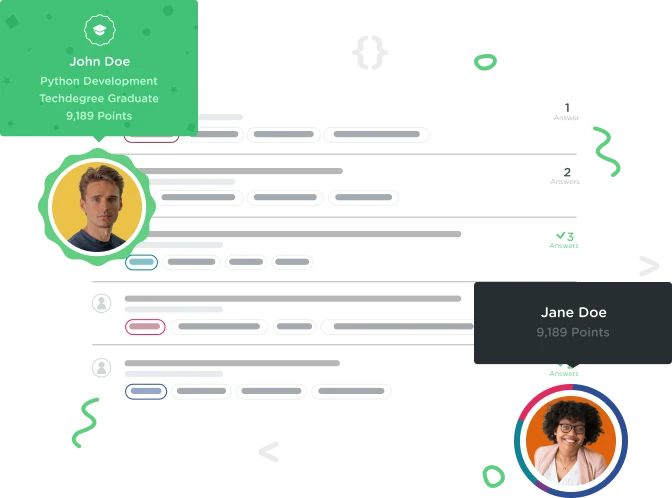

Joseph Carrillo
1,831 PointsHow to decrement using a for or while loop?
Hi Everyone,
I am new to Python programming. The code below works just fine but I want to decrement the initialized amount of tickets which is 100 until it hit zero tickets. For instance, below if you run my code, I enter the input of three; it outputs the difference of the initialized value of 100 and the result is 97 tickets. I want to loop so whenever the code runs it decrements until there are no more tickets.
Please see code below and your contributions or feedback is much appreciated.
#total tickets available
tickets_remaining = 100
#input from user
num_tickets = input("Hi customer! There are {} number of tickets left. How many tickets would you like to purchase? ".format(tickets_remaining))
#makes the input an integer
num_tickets = int(num_tickets)
#outputs the number of tickets they entered
print("You selected {} tickets you want to purchase.".format(num_tickets))
#prompts the user if this is the correct value that they entered
verify = input("Is this correct? Y/N? ")
#if the value is "Y" for yes then you take the initialized value minus the value the user inputs and output the remaining, else outputs another string.
if verify == "Y":
tickets_left = tickets_remaining - num_tickets
print("There are {} remaining tickets left. Thank you for your purchase.".format(tickets_left))
else :
print("Thank you for your inquiry. Have a great day!")
7 Answers

Christian Nogueras
298 PointsHmmm I don’t quite understand your question. You can create an while loop for example
while ticket_left > 0:
# Keep asking if they want to purchase more tickets
num_tickets = int(input("How many more ticket you want to purchase ? ")
ticket_left = ticket_left - num_tickets
exit_while_loop = str(input(“ Do you want to exist?. Enter ‘Y’ for yes and ‘N’ for no ”)
if exit_while_loop == Y:
break

Joseph Carrillo
1,831 PointsChristian Nogueras Thank you for your contributions and suggestions :)

Joseph Carrillo
1,831 PointsThank you in advance

Joseph Carrillo
1,831 PointsUpdate:
tickets_remaining = 100
num_tickets = input("Hi customer! There are {} number of tickets left. How many tickets would you like to purchase? ".format(tickets_remaining))
num_tickets = int(num_tickets)
print("You selected {} tickets you want to purchase.".format(num_tickets))
verify = input("Is this correct? Y/N? ")
while verify == "Y":
if tickets_remaining >= 0:
tickets_left = tickets_remaining - num_tickets
print("There are {} remaining tickets left. Thank you for your purchase.".format(tickets_left))
break
else :
print("Thank you for your inquiry. Have a great day!")

Joseph Carrillo
1,831 Pointstickets_remaining = 100
num_tickets = int(input("Hi customer! There are {} number of tickets left. How many tickets would you like to purchase? ".format(tickets_remaining)))
print("You selected {} tickets you want to purchase.".format(num_tickets))
if num_tickets == 0:
exit
else:
verify = input("Is this correct? Y/N? ")
if verify == "Y":
while (tickets_remaining >= num_tickets):
tickets_remaining = tickets_remaining - num_tickets
print("There are {} remaining tickets left. Thank you for your purchase.".format(tickets_remaining))
more_tickets = input("Would you like to purchase anymore tickets? Y/N? ")
if more_tickets == "Y":
num_tickets = num_tickets = int(input("How many more tickets would you like to purchase? ".format(tickets_remaining)))
else:
break

Joseph Carrillo
1,831 PointsSeems like I can achieve through repetitious if/else and nested if statements but that would clutter my code and require a lot of maintenance.

Joseph Carrillo
1,831 PointsChristian Nogueras does the code in my last post look correct?

Christian Nogueras
298 PointsI think that it would work for your purpose :)
Joseph Carrillo
1,831 PointsJoseph Carrillo
1,831 PointsThank you Christian. I wanted to make my code a slight different because I did not want to copy yours and find the root cause why code was executing the way I wanted it to. Last night, I was able to loop successfully but I could not get it to decrement correctly. I appreciate your efforts. I do want to try to accomplish using the for loop to use another method. I plan to use a function as well to condense my code but call on that function.