Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial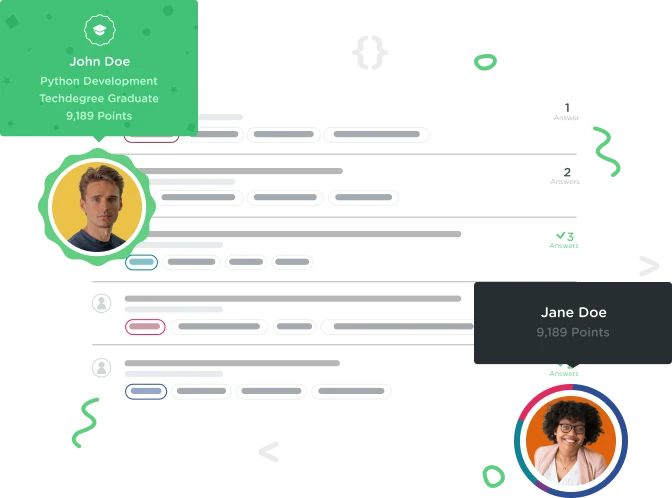

Xiaoxuan Wang
4,590 PointsHow to define propTypes in the component class if using ES6?
I coded the component class in the video in ES6:
class Counter extends React.Component {
render() {
return <div className="counter">
<button className="counter-action decrement"> - </button>
<div className="counter-score"> {this.props.score} </div>
<button className="counter-action increment"> + </button>
</div>
}
}
Unlike the example which Jim define propTypes inside the component class, but how shall I do if using ES6 syntax?
Thanks,
2 Answers

Luis Lopez
1,288 Pointsimport React, {Component} from 'react';
import PropTypes from 'prop-types';
class Counter extends Component {
static propTypes = {
score: PropTypes.number.isRequired
};
render() {
return (
<div className="player-score">
<div className="counter">
<button className="counter-action decrement ">-</button>
<div className="counter-score">{this.props.score}</div>
<button className="counter-action increment ">+</button>
</div>
</div>
);
}
}
This is how I wrote it using React v16 in my own editor utilizing create-react-app, I also had to npm install prop-types. Hope this helps anyone in a similar situation.
Xiaoxuan Wang
4,590 PointsXiaoxuan Wang
4,590 PointsThanks, Abraham
gevuong
2,377 Pointsgevuong
2,377 PointsIn React 15.5, PropTypes is extracted from the React object, and is its own separate package. This means you would need to install and import prop-types in your file. instead of using
React.PropTypes.number.isRequired
you use
PropTypes.number.isRequired
Here is an article on the topic: https://reactjs.org/blog/2017/04/07/react-v15.5.0.html#migrating-from-react.proptypes