Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial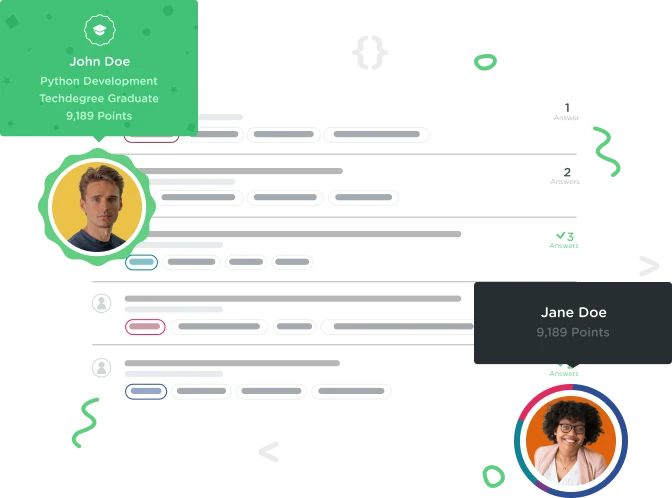

Abhishek Upadhyay
2,986 PointsHow to depict if the customer rep is serving a customer at the moment ?
I actually have couple of questions here :
Given :
- We have a queue of customer rep. Question : Is this a queue of available customer rep ?
- Each Customer rep has a list of customer it supported. Question : Got confused from the statement in Customer Rep class where we are adding the customer after the chat is ending ? Or Adding considering that is the start of the char,. I mean is it a list of customers each customer rep has supported or is supporting at the moment?
What I am trying to find is how to depict if a customer rep is free.
- Are we aiming to find it from the queue ( say the last one is the free one and the first one is still serving ) ?
- Or Is it determined from the assisted list of customers ( size = 0) something like that ?
5 Answers
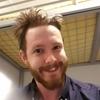
Chris Tvedt
3,795 PointsHeres the code with comments, i have left out some blanks with "CODE HERE"/"CONDITION HERE" tags for you to input the code yourself.
You got this!
public class CallCenter {
Queue<CustomerSupportRep> mSupportReps;
public CallCenter(Queue<CustomerSupportRep> queue) {
mSupportReps = queue; }
public void acceptCustomer(Customer customer) {
CustomerSupportRep csr = "CODE HERE" //first we poll() from the queue assigning a rep to the csr variable
/******************************************** *
* TODO (1) * Wait until there is an available rep in the queue.
* * While there is not one available, playHoldMusic
* * HINT: That while assignmentcheck loop syntax we used to
* * read files seems pretty similar
* ******************************************** */
/********************************************
* TODO (2)
* After we have assigned the rep, call the
* assist method and pass in the customer
********************************************
*/
/********************************************
* TODO (3)
* Since the customer support rep is done with
* assisting, put them back into the queue.
********************************************
*/
//if there where any reps in the queue, csr will now be assigned that rep, if not, csr == null. (TODO 1 == OK)
if ("CONDITION HERE" != null) {
//enters if statement and calls the assist method on csr (TODO 2 == OK)
"CODE HERE"
//When the assist method ends, the rep is placed back in the queue. (TODO 3 == OK)
"CODE HERE"
} else {
playHoldMusic();
}
}
public void playHoldMusic() {
System.out.println("Smooooooth Operator.....");
}
}
You will get this, if you're still struggling after this, i'll help explain the code even further, but give this a go, i'm sure you'll get it correct! :-)
Give me a shout if you need more help!
Please "Up-vote" the answers you find helpful :-)
Chris
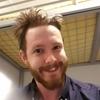
Chris Tvedt
3,795 PointsAnswers follow in bold:
Given :
We have a queue of customer rep. Question : Is this a queue of available customer rep ? They are asking you to make a queue of available reps yes, once the rep has be assigned to a costumer, the rep should be taken out of the queue
Each Customer rep has a list of customer it supported. Question : Got confused from the statement in Customer Rep class where we are adding the customer after the chat is ending ? Or Adding considering that is the start of the char,. I mean is it a list of customers each customer rep has supported or is supporting at the moment? The list is supposed to be costumers the rep HAS supported
What I am trying to find is how to depict if a customer rep is free.
Are we aiming to find it from the queue ( say the last one is the free one and the first one is still serving ) ? Or Is it determined from the assisted list of customers ( size = 0) something like that ? Use the Queue(click to see the API) class to make a queue, that way, you can use .poll() to take the costumer rep from the top of the queue every time and the .add() method to add them back in at the back of the queue when they are finished with the costumer
Hope this helps you understand the task better. Up-vote if it did please :-)

Abhishek Upadhyay
2,986 PointsThanks Chris.
I tried to run it but still having some problems which I am sure u can figure out in a second.
{code} import java.util.ArrayDeque; import java.util.Queue;
public class CallCenter { Queue<CustomerSupportRep> mSupportReps;
public CallCenter(Queue<CustomerSupportRep> queue) { mSupportReps = queue; }
public void acceptCustomer(Customer customer) { CustomerSupportRep csr; /******************************************** * TODO (1) * Wait until there is an available rep in the queue. * While there is not one available, playHoldMusic * HINT: That while assignmentcheck loop syntax we used to * read files seems pretty similar ******************************************** */
boolean repsAvailable=mSupportReps.poll()!=null;
while(repsAvailable) {
csr = mSupportReps.poll();
csr.assist(customer);
if (csr.getAssistedCustomers().contains(customer)) {
mSupportReps.add(csr);
}
else {
System.out.printf ("%s not found",customer.getName());
}
if(!repsAvailable) {
playHoldMusic();
break;
}
}
/********************************************
* TODO (2)
* After we have assigned the rep, call the
* assist method and pass in the customer
********************************************
*/
/********************************************
* TODO (3)
* Since the customer support rep is done with
* assisting, put them back into the queue.
********************************************
*/
}
public void playHoldMusic() { System.out.println("Smooooooth Operator....."); }
}
{code}

Abhishek Upadhyay
2,986 PointsThanks Chris I have done this as below . However, I think the way the question has been framed to use a loop is not a clear requirement here though and confuses. The below solution uses no Loop . I hope you understand.
Many Thanks again and I have upvoted your answer.
{code} import java.util.ArrayDeque; import java.util.Queue;
public class CallCenter { Queue<CustomerSupportRep> mSupportReps;
public CallCenter(Queue<CustomerSupportRep> queue) { mSupportReps = queue; }
public void acceptCustomer(Customer customer) { CustomerSupportRep csr = mSupportReps.poll(); /******************************************** * TODO (1) * Wait until there is an available rep in the queue. * While there is not one available, playHoldMusic * HINT: That while assignmentcheck loop syntax we used to * read files seems pretty similar ******************************************** */
/********************************************
* TODO (2)
* After we have assigned the rep, call the
* assist method and pass in the customer
********************************************
*/
/********************************************
* TODO (3)
* Since the customer support rep is done with
* assisting, put them back into the queue.
********************************************
*/
//if there where any reps in the queue, csr will now be assigned that rep, if not, csr == null. (TODO 1 == OK)
if (mSupportReps.poll() != null) {
//enters if statement and calls the assist method on csr (TODO 2 == OK)
csr.assist(customer);
//When the assist method ends, the rep is placed back in the queue. (TODO 3 == OK)
mSupportReps.add(csr);
} else {
playHoldMusic();
}
}
public void playHoldMusic() { System.out.println("Smooooooth Operator....."); }
}
{code}
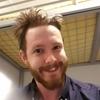
Chris Tvedt
3,795 PointsThe loop you think of will be initiated in the "main"-method. Implementing a loop in the function will not be viable since it then will need to be called manually in the main method again every time the "acceptCostumer()" method ends. Looping in the "main"-method and not in the "acceptCostumer"-method is, in my opinion, the best solution. And will get you to pass the challenge.
And btw, in the "if"-statement i would swap the "mSupportReps.poll()" to "csr" since csr == mSupportReps.poll(). But glad i could help you out and get the challenge passed.
Please let me know if there is anything, and i will try my best to help you :-)

Abhishek Upadhyay
2,986 PointsThanks Chris , I think it should have been mentioned in the task definition to make things clearer but I am glad you were able to help me out which I am so thankful again !