Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial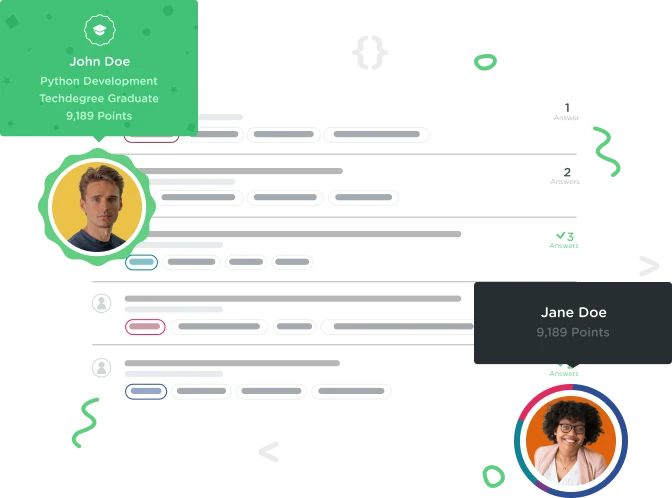

Amardeep Thadisetty
1,347 Pointshow to display alert box in login screen in php if a user enter's a wrong password. I have tried that in ajax. Is there
Is there any way to do it. when a user enter's a corret username and password we let him in. IF he don't how to achieve it?
3 Answers

Jeremy Hayden
1,740 PointsPhp is server side script, meaning its done once the website hits the users browser.
Pop up windows are usually done with a client side script like javascript.
You can either code the javascript directly into your html, or make it dynamic by echoing it out of php. But php is not making the pop up, javascript is.
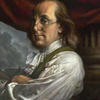
Jeff Busch
19,287 PointsHi Amardeep,
Here is a simple example:
<?php
/*****
* Query database to retrieve stored
* username and password then check validity below
*****/
$Query_username = "something1";
$Query_pswd = "something2";
$userName = $_POST['userName'];
$pswd = $_POST['pswd'];
if(($userName == $Query_username) && ($pswd == $Query_pswd)) {
echo "OK, logged in, good to go.";
} else {
$message = "Username and/or Password incorrect.\\nTry again.";
echo "<script type='text/javascript'>alert('$message');</script>";
}
?>
Jeff
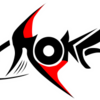
Justin Black
24,793 Pointsto do it in javascript, you'd want to submit the entire form using javascript....
<script type="text/javascript">
$(document).on('click', '.formsubmitbutton', function(e) {
e.preventDefault();
$.ajax({
type: "POST",
url: $(".form").attr('action'),
data: $(".form").serialize(),
success: function(response) {
if (response === "success") {
window.reload;
} else {
alert("invalid username/password. Please try again");
}
}
});
});
</script>
Now that is a very basic example, but would function.. The PHP is pretty simple... check database, to see if the username and password exist and match... if not echo "error"; if they do echo "success"