Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial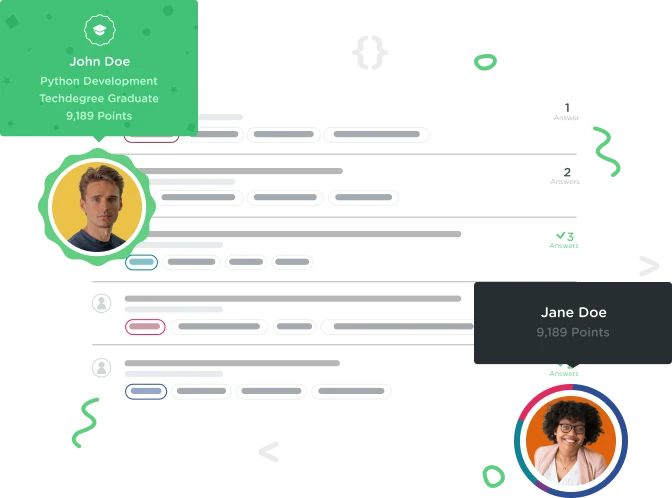
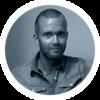
Daniel Fitzhugh
9,715 PointsHow to Display Photos using Regular Javascript and JSONP
I don't like learning stuff in jQuery without knowing how to do it regular javascript.... so I read a couple of articles and worked this out.
I managed to replicate the basic functionality.... but I wasn't able to dynamically generate the URL Query String on the fly, as it had already been defined prior to the event listener being executed (see code comments below).
Does anyone know how to structure this so that the Query String could be generated dynamically ?
// CODE FOR JSONP USING REGULAR JAVSCRIPT
// IT ADDS A <head> TAG TO THE DOM CONTAINING THE QUERY STRING "jsoncallback=jsonFlickrFeed"
// "jsonFlickrFeed" IS A FUNCTION CALL THAT THE ACTUAL JSON FILE IS WRAPPED IN (if you look at it)
const script = document.createElement("script");
script.type = "text/javascript";
script.src = "http://api.flickr.com/services/feeds/photos_public.gne?jsoncallback=jsonFlickrFeed&format=json";
document.getElementsByTagName("head")[0].appendChild(script);
// WHEN THE SCRIPT TAG HAS BEEN ADDED, A FUNCTION WITH THE NAME "jsonFlickrFeed" IS EXECUTED AUTOMATICALLY
// THE "data" ARGUMENT IS THE PARSED JSON DATA --- AND YOU CAN ONLY USE IT WITHIN THIS FUNCTION
// THUS I HAVE PLACED THE EVENT LISTENER WITHIN THIS FUNCTION
// HOWEVER THE EVENT LISTENER CAN'T DICTATE WHAT THE QUERY STRING IS NOW, BECAUSE IT IS EXECUTED AFTER THE QUERY STRING HAS ALREADY BEEN DEFINED
function jsonFlickrFeed(data) {
const ulButtons = document.querySelector(".filter-select");
const buttons = document.querySelectorAll("button");
const photosArray = data.items;
ulButtons.addEventListener("click", function(event) {
console.log(photosArray);
for ( let i = 0; i < buttons.length; i += 1 ) {
buttons[i].className = "";
}
if ( event.target.tagName === "BUTTON" ) {
event.target.className = "selected";
}
const div = document.querySelector("#photos");
const ul = document.createElement("ul");
div.appendChild(ul);
for ( i = 0; i < photosArray.length; i += 1 ) {
const photo = photosArray[i];
const li = document.createElement("li");
li.className = "grid-25 tablet-grid-50";
const a = document.createElement("a");
a.setAttribute("href", photo.link);
a.className = "image";
const img = document.createElement("img");
img.setAttribute("src", photo.media.m);
ul.appendChild(li);
li.appendChild(a);
a.appendChild(img);
}
});
};
ps. I have no intention of using regular javascript for this in the future --- writing it is a nightmare and I'm sure it's really bad security-wise ;)