Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial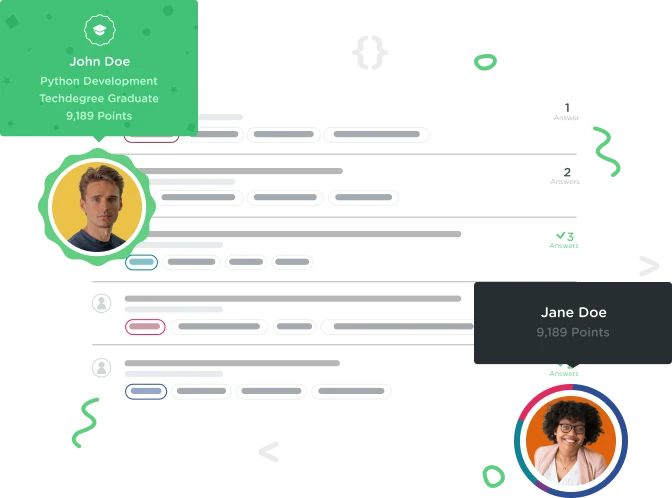

Minseok Kim
2,708 Pointshow to do the challenge?
def word_count(string):
string = string.lower().split()
dictionary = {}
for a in string:
dictionary[a] = string.count(a)
return dictionary
What's wrong? I see people doing += 1, but I don't know how that would work either..

Minseok Kim
2,708 Points
Tanja Riet
10,803 Points# my solution: create an empty dictionary and fill it
[MOD: we don't provide unexplained solutions; sorry - srh]
2 Answers

Øyvind Andreassen
16,839 PointsHey,
Looking at your solution it actually works. More elegant than the solution that I came up with! It also passes the challenge when I try it, so I would try it again and look out for pesky whitespaces that will mess up your indentation. If tabs isn't working try doing manual four spaces for each indentation level.
If it still doesn't pass this is my solution. That also passes.
def word_count(string):
dictionary = {}
word_list = string.lower().split()
for word in word_list:
if word in dictionary:
dictionary[word] += 1
else:
dictionary[word] = 1
return dictionary
Simple loop through each word in the list and counts how many times it comes up.
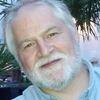
Jeff Muday
Treehouse Moderator 28,717 PointsYour approach is pretty good. The cool thing about Python and... well almost any other language is there are MANY ways to do things. I show you three different ways to solve it below.
A big issue is that if you attempt to access a dictionary key that DOES NOT exist, Python will throw an error. So you have three workarounds (I am sure there are other good ways to do this too!)
This is the simplest approach-- the GET method on the dictionary object
def word_count(string):
string = string.lower().split()
dictionary = {}
for a in string:
dictionary[a] = dictionary.get(a,0) + 1
return dictionary
Using dictionary with key/values that have been "pre-zeroed". The extra loop makes this slightly slower.
def word_count(string):
string = string.lower().split()
dictionary = {}
for a in string:
dictionary[a] = 0
for a in string:
dictionary[a] += 1
return dictionary
Using the TRY/EXCEPT block (try/except is a little slow)
def word_count(string):
string = string.lower().split()
dictionary = {}
for a in string:
try:
dictionary[a] += 1
except:
dictionary[a] = 1
return dictionary
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsHave you got a link to the challenge, please?