Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial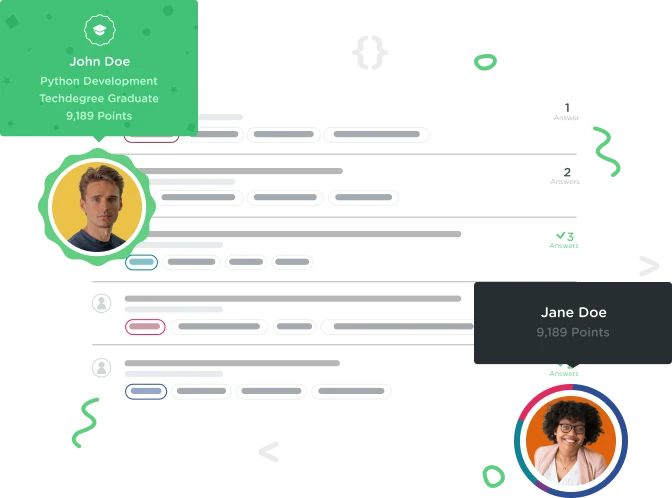

serena chan
iOS Development Techdegree Student 3,606 PointsHow to ensure the number of experienced players are the same for each team, in the soccer league coordinator project.
I've been stuck for a long time on how to add the players into each team, in a way that each team has the amount of numberOfExperiencedPlayers/3.
Here is the code I have right now:
let playerName : [Int: String] = [
1: "Joe Smith",
2: "Jill Tanner",
3: "Bill Bon",
4: "Eva Gordon",
5: "Matt Gill",
6: "Kimmy Stein",
7: "Sammy Adams",
8: "Karl Saygan",
9: "Suzane Greenberg",
10: "Sal Dali",
11: "Joe Kavalier",
12: "Ben Finkelstein",
13: "Diego Soto",
14: "Chloe Alaska",
15: "Arnold Willis",
16: "Phillip Helm",
17: "Les Clay",
18: "Herschel Krustofski"
]
let playerHeight : [Int: Int] = [
1: 42,
2: 36,
3: 43,
4: 45,
5: 40,
6: 41,
7: 45,
8: 42,
9: 44,
10: 41,
11: 39,
12: 44,
13: 41,
14: 47,
15: 43,
16: 44,
17: 42,
18: 45
]
//true for YES, false for NO
let playerSoccerExp : [Int: Bool] = [
1: true,
2: true,
3: true,
4: false,
5: false,
6: false,
7: false,
8: true,
9: true,
10:false,
11:false,
12: false,
13: true,
14: false,
15: false,
16: true,
17: true,
18: true
]
let playerGuardianName : [Int: String] = [
1: "Jim and Jan Smith",
2: "Clara Tanner",
3: "Sara and Jenny Bon",
4: "Wendy and Mike Gordon",
5: "Charles and Sylvia Gill",
6: "Bill and Hillary Stein",
7: "Jeff Adams",
8: "Heather Bledsoe",
9: "Henrietta Dumas",
10: "Gala Dali",
11: "Sam and Elaine Kavalier",
12: "Aaron and Jill Finkelstein",
13: "Robin and Sarika Soto",
14: "David and Jamie Alaska",
15: "Claire Willis",
16: "Thomas Helm and Eva Jones",
17: "Wynonna Brown",
18: "Hyman and Rachel Krustofski"
]
/* Part 2*/
//Each team is modeled by a dictionary storing players' index and experience status
var teamDragons : [Int: Bool]
var teamSharks : [Int: Bool]
var teamRaptors : [Int: Bool]
//ensures players are distributed evenly; all teams will have same number of players
let totalPlayerInTeam = playerSoccerExp.count/3
//counter for the number of experienced players
var numExpPlayers = 0
for (index,experience) in playerSoccerExp {
if(experience == true){
++numExpPlayers
}
}
//number of experienced players per team, assuming there are 3 teams
var expPlayersPerTeam = numExpPlayers/3
//temp playerSoccerExp
var tempPlayerSoccerExp = playerSoccerExp
//ensures number of experienced players and number of players are the same for each team
for(index,experience) in tempPlayerSoccerExp{
while(teamDragons.count < totalPlayerInTeam){
teamDragons[index] = experience //filling up 1st [Int: Bool]
tempPlayerSoccerExp.removeValueForKey(index)
//if teamDragon's number of true boolean >= expPlayerPerTeam, stop
As you can see, the method I have used in for(index,experience) in tempPlayerSoccerExp, has a couple of problems because I can't use //if teamDragon's number of true boolean >= expPlayerPerTeam, stop.
That's because, what if the if statement is never false? Say if the player's were arranged where the first 6 players had 'false' in playerSocExp, or no experience. Then 6 players with no experience will be added into teamDragons, which won't help in equal distribution of experienced players.
Much help appreciated!
4 Answers
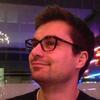
Alex Millius
iOS Development Techdegree Student 5,468 PointsHi Serena,
You made a great job at naming your variables and commenting your code!
There is something that immediately catch my eye however. It looks like you forgot an important concept of a dictionary collection. As explain in the documentation, "Dictionaries are UNORDERED collections of key-value associations. " CollectionType
This means, you can't make different dictionary for the name, experience, guardian etc.. of a player. Dictionary doesn't function like an array, there is no specific order, only key-value pair.
Maybe you could try to make a dictionary for a single player? Something like: serenaChan = ["name" : "Serena Chan", "favouriteNumber" : 7, "loveSunnyDay" : true] Swift will automatically extrapolate the type of your dictionary ;)
If you extrapolate the phrase "Programs must be written for people to read, and only incidentally for machines to execute.", you can ask yourself, "How would I do if I had 18 kids in front of me ?"
I think you'll say something like "Ok guys, if you have already play soccer, go on my right, if you haven't already play soccer, go on my left"
I don't want to give you the answer straight away :)
Your code looks a bit over complicated. Take a fresh look at the instructions. You should use only "simple" stuff.
- Accessing, appending and counting Arrays
- Accessing Dictionaries and Arrays
So there is no need of ".removeValueForKEy()"
I think I said enough, good luck :)

serena chan
iOS Development Techdegree Student 3,606 PointsHi,
Thanks for your response :) Even though dictionaries are unordered, I can iterate through the entire dictionary and sort/filter the entries into an experienced player array, or non experienced player array accordingly. Here is my final solution:
By the way, have you started the second project? I happen to be stuck in adding constraints, that makes the proportions and sizes of elements constant in different sized iPhone screens.
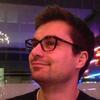
Alex Millius
iOS Development Techdegree Student 5,468 PointsHi,
I give you that, you can iterate through dictionaries and keep track of the indexes.
But unfortunately you've got several bugs and missing part in your code :-/
Part 1: "In addition, create an empty collection variable to hold all the players’ data" I don't see that in your code. you need an empty collection variable for all the players, not only for the three teams.
"Please choose an appropriate data type to store the information for each player" What you did is "Please choose appropriateS data typeS to store the information for each player" You have three different data set to store a single player. Even if it was correct from an instruction perspective, your data are spread all around, you could easily make a typo.
Part2: "(...)and should work, if we theoretically had more or less than 18 players, (...)" With your solution -> let totalPlayerInTeam = playerSoccerExp.count/3, your code doesn't work if we have a number of player that have a rest when divided by 3. If you have 19 and 20 player for example, your code will always have a totalPlayerInTeam of 6.
Part3: Your code give letters like this: "Optional("Wynona Brown"), Your child Optional("Les Clay") from Team Dragon will attend their first team practice on March 17, 1pm" You have to get rid of the "Optional("")"part ( you could also use the empty collection variable created in part 1 to avoid duplicating your code three times)
I hope it help
For the second project, you don't need to keep the proportions and sizes of elements. "add constraints to maintain the layout such all UI elements are sized and spaced appropriately for all iPhones 3, 4, 5, 6 and 6+." You just need to be sized and spaced appropriately. You dont have to make a masterpiece ;) The trick is not to try to format the size of every elements, but to format the distance between the elements and the bounds of the view :) Let your buttons and label live their lives and take the space they want.
bye

Kenneth Dubroff
10,612 PointsThis also appends the same players to each team