Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial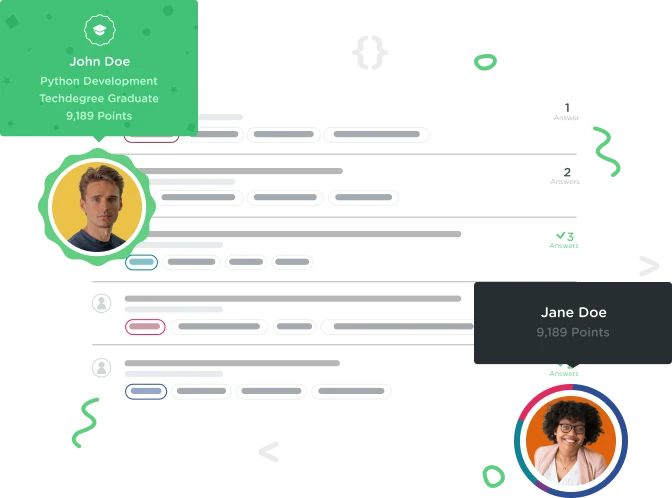

iwgpnjvycw
8,577 PointsHow to execute external API calls with React + Express
Hi everyone,
I'm still fairly new to full stack development and I've been stuck for days. I could really use some help from anyone who is familiar with React + Express + External API projects. Right now I still have my frontend and backend working independently of each other, but I'm at the stage where I need to start wiring up the frontend to the backend.
I have a file (PhotosAPI.js
) with the following code on the React side:
const API = 'https://api.unsplash.com/';
export const getCurated = () =>
fetch(`${API}photos/curated/?order_by=popular&client_id=${API_KEY}`).then(
res => res.json()
);
export const search = query =>
fetch(`${API}search/photos?query=${query}&client_id=${API_KEY}`)
.then(res => res.json())
.then(data => data.results);
Then in App.js I make the fetch
request in componentDidMount()
and set the state like so:
componentDidMount() {
PhotosAPI.getCurated()
.then(photos => {
this.setState({
photos,
loading: false
});
})
.catch(error => this.setState({ error, loading: false }));
}
My question isββnow that I'm trying to integrate the backend--how should the fetch
request be handled? Do I need to move PhotosAPI.js
to the backend? If yes, then how do I pass the response back to componentDidMount()
in the frontend so that it sets the state properly?
Any guidance on how to properly set this process up is greatly appreciated!