Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial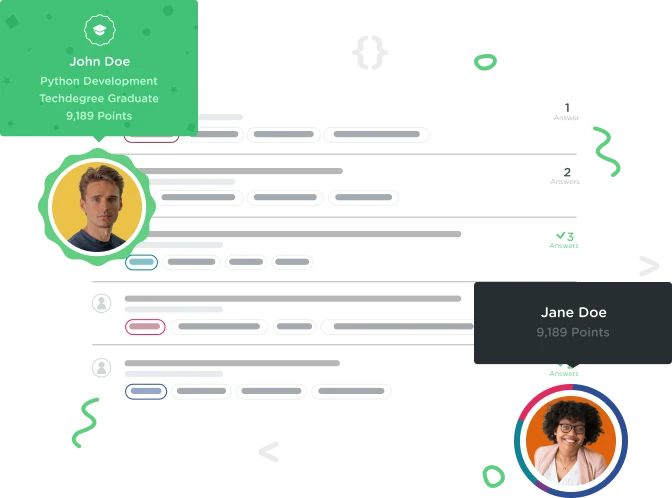
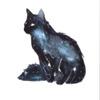
Antony .
2,824 PointsHow to find a specific word in a string and place it in a variable?
I need to find the word "Antony" inside this array and place it in a global variable.
let word = ["Hi my name is Antony and I like to code!"];
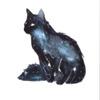
Antony .
2,824 Pointsupdated my post!
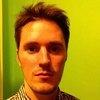
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsIs the string always going to be in this format? Like:
const introductions = [`Hi my name is Antony and I like to code!"`, `Hi my name is Brendan and I like to code!`]
1 Answer
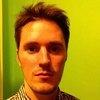
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsOne way of solving this is with the split method on strings. We have this string "Hi my name is Antony and I like to code!"
. We can call .split()
on it passing in an argument for how to split the string. So we can pass in "Hi my name is " and it will split the string by that separator. We end up with an array of two items, the first one being just an empty string:
const intro = "Hi my name is Antony and I like to code!"
const remainder = intro.split("Hi my name is ") // [ '', 'Antony and I like to code!' ];
We can grab the 2nd item (index 1) in that array, and then call split
on it again this time passing in " and I like to code!". When we get an array back from this call to split
, the name we're looking for will be at index 0 because it should be right at the beginning:
const name = remainder[1].split(" and I like to code!")[0]; // 'Antony'
We can put all of this in a function, and then map over an array to get all the names:
const introductions = [
"Hi my name is Antony and I like to code!",
"Hi my name is Brendan and I like to code!"
]
const findNameFromString = (str) => {
return str
.split("Hi my name is ")[1]
.split(" and I like to code!")[0];
}
const names = introductions.map(findNameFromString); // [ 'Antony', 'Brendan' ]
An alternative could be to use a regular expression.
const regexFindNameFromString = (str) => {
const regex = /Hi my name is (?<name>[\w\s]+) and I like to code!/;
return regex.exec(str).groups.name;
}
const names = introductions.map(regexFindNameFromString); // [ 'Antony', 'Brendan' ]
There are probably other ways too.
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsBrendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsCan you give a little more context? Can you give an example of the string and what word you need to find in it?