Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial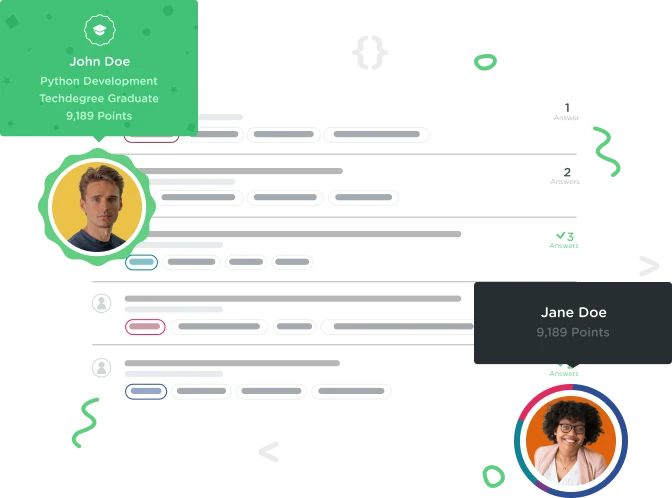
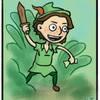
Rebecca Jensen
11,580 PointsHow to find matching values in array, then choose the larger index value
I'm working on a challenge (strictly for my own practice) to look at an array of "credit card numbers" (strings of 16 digits, with dashes), add up each string, choose the greatest value, then return the credit card number of the greatest value. I have been able to get this far!
However, the last step is to handle the case when there are two (or more) numbers that are equal, when added up. In this situation, I should return the one that appears later in the array.
My approach is to:
- check that there is a match
- if there is a match, compare the index values
- choose the number at the greater index value
I'm stuck on 2 things:
- How to cycle over an array and find a match, without matching the value to itself
- Calling the matched values out, and comparing their index values
Any thoughts on what methods I should use?
Code on repl.it: https://repl.it/I0e5/26
var ccNumbers = ['1111','888-222', '21-11', '9989', '9918'];
function addDigits(number){
var sum = 0; //store the sum
var sumArray = [];//store several sums to print
for ( x = 0; x < ccNumbers.length; x++ ) { //loop through ccNumbers array
var ccNmbr = ccNumbers[x]; //pick out one array item and store it in ccNmbr
for ( z = 0; z < ccNmbr.length; z++) {
ccNmbr = ccNmbr.replace("-", 0);
ccNmbr = ccNmbr.replace(" ", 0);
} //removes dashes, replaces with 0's, to avoid NaN
for ( y = 0; y < ccNmbr.length; y++ ) { //loop through each digit in ccNmbr
sum += parseInt(ccNmbr.charAt(y), 10); //add each digit to the sum
} //close adding digits loop
sumArray.push(sum); //add sum to an array
sum = 0; //reset sum to 0 for the next loop
} //close looping through array loop
var largest = Math.max(...sumArray); //finds the largest added up number
for ( i = 0; i < sumArray.length; i++ ) {
if ( largest === sumArray[i]) { //if there is a match... This isn't working. It matches itself.
largest = Math.max( 2, 5);//choose the greater index value?? Values here just for testing.
}
}
return largest;
//var position = sumArray.indexOf(largest); //finds its position in the array
//return position;
//var largestCC = ccNumbers[position]; //find the corresponding CC number at same position
//return largestCC;
}
addDigits();
2 Answers

Stephan Olsen
6,650 PointsI hope I understood you correctly. If you want the index of the largest number, you can simply store the value of i in a variable, and use that as index on your ccNumbers array.
let index = 0;
for ( i = 0; i < sumArray.length; i++ ) {
if ( largest === sumArray[i]) { //if there is a match... This isn't working. It matches itself.
index = i;
}
}
ccNumbers[index]; // '9989'
Hope it makes sense, otherwise let me know :)
By the way, if you want some suggestions on how to improve your code, I have a few.
If you declare the sum variable inside the outer for loop, you wont have to reset the sum.
You're also adding a parameter to your function, but not using it anywhere inside the function. For reusability I would definetely suggest you make use of this.
I created as jsfiddle with the improvements if you're curious to see, otherwise good job! https://jsfiddle.net/67scdj2j/

Joe Beltramo
Courses Plus Student 22,191 PointsUnless you use a regex to find the thing to replace, removing the character loop won't work...It does for single spaces and/or hyphens but the minute there is more than one hyper or space, like in a credit card number, you will get an unexpected result.
Just to let you know :]

Stephan Olsen
6,650 PointsAhh, did not know that, my bad. Fixed my post now :)
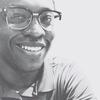
Ricardo Hill-Henry
38,442 Pointslet greatest = Math.max(...sumArray), //retrieves the greatest numerical value in the array (it's index isn't important here)
card = ccNumbers[sumArray.indexOf(greatest)]; /* indexOf() retrieves the index of the first match, then we use that to get the cc since the arrays values correspond in the two arrays */
Joe Beltramo
Courses Plus Student 22,191 PointsJoe Beltramo
Courses Plus Student 22,191 PointsWhat is the intended goal here? Are you trying to return the
largest
credit card number as which one has the greatest sum?So in your example:
Therefore you are expecting to return '9989'?