Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial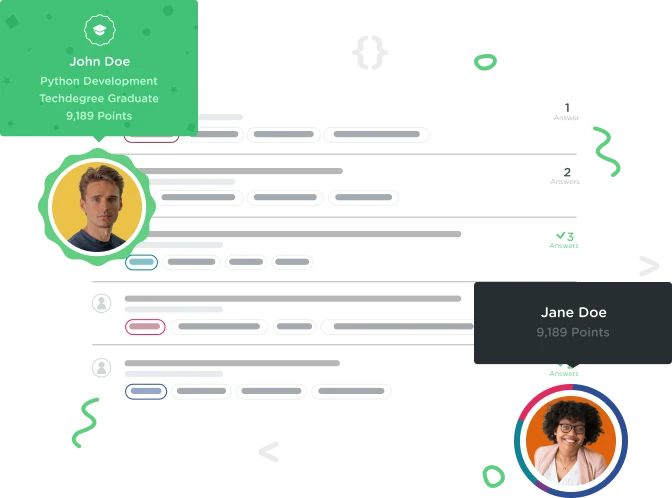

Fabiola Barrientos
9,467 Pointshow to finish loop
I feel like I am close to finishing this but simply cannot figure out how to complete this loop. A little bit of help would be appreaciated.
namespace Treehouse.CodeChallenges
{
class FrogStats
{
public static double GetAverageTongueLength(Frog[] frogs)
{
double sum = 0;
double i = 0;
for(; i < frogs.Length; i++ )
{
sum += Frog.TongueLength;
}
i++;
return sum / i;
}
}
}
namespace Treehouse.CodeChallenges
{
public class Frog
{
public int TongueLength { get; }
public Frog(int tongueLength)
{
TongueLength = tongueLength;
}
}
}
1 Answer
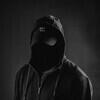
Tim Danner
3,284 PointsFirst, you're accessing the class directly instead of its instances, and second, you're assigning the values of type int
to the variable sum
of type double
.
The solutions would be to iterate through the Frog
array that's provided in the parameter, and to cast TongueLength
to double
:
namespace Treehouse.CodeChallenges
{
class FrogStats
{
public static double GetAverageTongueLength(Frog[] frogs)
{
double sum = 0;
double i = 0;
for (; i < frogs.Length; i++)
{
// explicit cast by appending (double)
sum += (double)frogs[i].TongueLength;
}
i++;
return sum / i;
}
}
}
While the solution above is legit, the codes could look a little bit confusing to other coders.
I'd suggest keeping i
inside the for loop because it doesn't have any specific meaning outside the scope, and casting sum
to double
at the end of the calculation for better performance:
namespace Treehouse.CodeChallenges
{
class FrogStats
{
public static double GetAverageTongueLength(Frog[] frogs)
{
// double sum = 0;
// double i = 0;
int sum = 0;
for (int i = 0; i < frogs.Length; i++)
{
sum += frogs[i].TongueLength;
}
// i++;
return (double)sum / frogs.Length;
}
}
}
In C#, when it comes to division, as long as either side is of type double
, the return value will always be double
.
You can verify this by calling the System
method GetType()
:
Console.WriteLine((5.0/2).GetType());